编写顺序表的初始化、插入、删除和查找算法
时间: 2023-05-30 16:06:06 浏览: 142
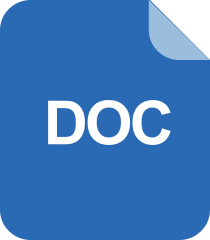
顺序表的初始化插入删除等基本操作算法(C语言).doc
1. 初始化顺序表
```
void InitList(SeqList &L)
{
L.elem = (ElemType*)malloc(MAXSIZE * sizeof(ElemType)); // 动态分配存储空间
if(!L.elem) exit(1); // 分配失败,退出程序
L.length = 0; // 初始化长度为0
}
```
2. 插入元素
```
bool ListInsert(SeqList &L, int i, ElemType e)
{
if(i < 1 || i > L.length + 1) return false; // 插入位置不合法
if(L.length >= MAXSIZE) return false; // 顺序表已满
for(int j = L.length; j >= i; j--)
{
L.elem[j] = L.elem[j - 1]; // 将第i个位置及其后面的元素后移
}
L.elem[i - 1] = e; // 插入元素e
L.length++; // 长度加1
return true;
}
```
3. 删除元素
```
bool ListDelete(SeqList &L, int i, ElemType &e)
{
if(i < 1 || i > L.length) return false; // 删除位置不合法
e = L.elem[i - 1]; // 取出要删除的元素
for(int j = i; j < L.length; j++)
{
L.elem[j - 1] = L.elem[j]; // 将第i个位置及其后面的元素前移
}
L.length--; // 长度减1
return true;
}
```
4. 查找元素
```
int LocateElem(SeqList L, ElemType e)
{
for(int i = 0; i < L.length; i++)
{
if(L.elem[i] == e) return i + 1; // 找到元素,返回位置
}
return 0; // 没找到元素,返回0
}
```
阅读全文
相关推荐


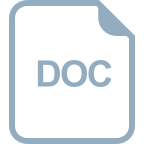


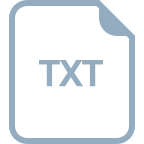
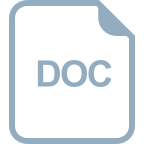
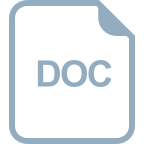







