在unity做一个烟花,用ECS框架,附代码
时间: 2023-12-03 22:42:12 浏览: 77
下面是一个简单的使用Unity ECS框架创建烟花的示例代码:
```csharp
using Unity.Entities;
using Unity.Transforms;
using Unity.Collections;
using Unity.Mathematics;
using UnityEngine;
public class FireworkManager : MonoBehaviour
{
public GameObject fireworkPrefab;
private EntityManager entityManager;
private Entity fireworkEntity;
private void Start()
{
entityManager = World.Active.EntityManager;
// 创建烟花实体
fireworkEntity = entityManager.CreateEntity(
typeof(Translation),
typeof(Velocity),
typeof(Firework)
);
}
private void Update()
{
if (Input.GetMouseButtonDown(0))
{
// 创建烟花
CreateFirework();
}
// 更新烟花位置和速度
Entities.ForEach((ref Translation translation, ref Velocity velocity) =>
{
translation.Value += velocity.Value * Time.deltaTime;
velocity.Value += new float3(0, -9.81f, 0) * Time.deltaTime;
});
}
private void CreateFirework()
{
// 获取烟花的初始位置
var mousePos = Input.mousePosition;
mousePos.z = Camera.main.transform.position.y;
var position = Camera.main.ScreenToWorldPoint(mousePos);
position.z = 0;
// 在实体管理器中添加烟花组件
entityManager.AddComponentData(fireworkEntity, new Translation { Value = position });
entityManager.AddComponentData(fireworkEntity, new Velocity { Value = new float3(UnityEngine.Random.Range(-5f, 5f), UnityEngine.Random.Range(10f, 20f), 0) });
entityManager.AddComponentData(fireworkEntity, new Firework { ExplosionTime = UnityEngine.Random.Range(1f, 2f) });
// 在场景中创建烟花游戏对象
var fireworkObject = Instantiate(fireworkPrefab, position, Quaternion.identity);
entityManager.AddSharedComponentData(fireworkEntity, new RenderMesh { mesh = fireworkObject.GetComponent<MeshFilter>().sharedMesh, material = fireworkObject.GetComponent<MeshRenderer>().sharedMaterial });
}
}
public struct Velocity : IComponentData
{
public float3 Value;
}
public struct Firework : IComponentData
{
public float ExplosionTime;
}
```
这个示例代码中,我们首先在`Start`方法中创建了一个烟花实体,并在`Update`方法中更新所有烟花的位置和速度。当玩家点击鼠标左键时,我们会调用`CreateFirework`方法来创建一个新的烟花实体。
在`CreateFirework`方法中,我们首先获取了鼠标点击的位置,并将其转换为世界坐标系中的位置。然后,我们向烟花实体添加了三个组件:`Translation`表示烟花的位置,`Velocity`表示烟花的速度,`Firework`表示烟花爆炸的时间。最后,我们在场景中创建了一个烟花游戏对象,并将其网格和材质分别附加到烟花实体上。
需要注意的是,这个示例代码只是一个简单的演示,你可以根据自己的需要对其进行修改和扩展。
阅读全文
相关推荐
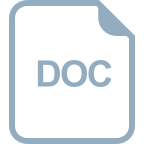
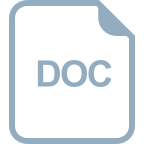
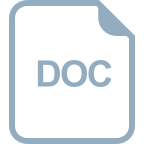






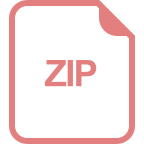
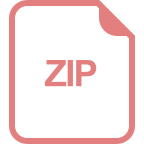
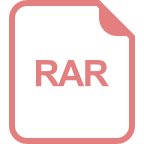
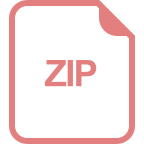
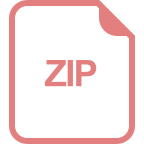
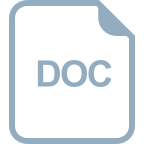



