给我用JS编写一个扫雷的游戏代码
时间: 2023-09-10 08:05:17 浏览: 94
### 回答1:
当然可以!以下是一份简单的 JavaScript 代码,实现了扫雷游戏的基本功能:
```
const width = 9;
const height = 9;
const bombs = 10;
let board = [];
let revealed = [];
// 初始化棋盘
for (let i = 0; i < height; i++) {
board[i] = [];
revealed[i] = [];
for (let j = 0; j < width; j++) {
board[i][j] = 0;
revealed[i][j] = false;
}
}
// 随机放置地雷
let placedBombs = 0;
while (placedBombs < bombs) {
let x = Math.floor(Math.random() * width);
let y = Math.floor(Math.random() * height);
if (board[y][x] !== "bomb") {
board[y][x] = "bomb";
placedBombs++;
}
}
// 计算每个格子周围地雷数量
for (let i = 0; i < height; i++) {
for (let j = 0; j < width; j++) {
if (board[i][j] === "bomb") {
continue;
}
let bombCount = 0;
for (let yoffset = -1; yoffset <= 1; yoffset++) {
for (let xoffset = -1; xoffset <= 1; xoffset++) {
let y = i + yoffset;
let x = j + xoffset;
if (y >= 0 && y < height && x >= 0 && x < width && board[y][x] === "bomb") {
bombCount++;
}
}
}
board[i][j] = bombCount;
}
}
// 点击事件处理函数
function onClick(x, y) {
if (revealed[y][x]) {
return;
}
revealed[y][x] = true;
if (board[y][x] === "bomb") {
alert("你踩到地雷了!游戏结束。");
} else {
revealSurrounding(x, y);
}
}
// 展开周围格子
function revealSurrounding(x, y) {
for (let yoffset = -1; yoffset <= 1; yoffset++) {
for (let xoffset = -1; xoffset <= 1; xoffset++) {
let y2 = y + yoffset;
let x2 = x + xoffset;
if (y2 >= 0 && y2 < height && x2 >= 0 && x2 < width && !revealed[y2][x2]) {
revealed[y2][x2] =
### 回答2:
扫雷是一款经典的益智游戏,下面是一个用JS编写的简单版本扫雷游戏的代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>扫雷游戏</title>
<style>
.container {
display: inline-block;
margin-bottom: 10px;
}
.cell {
display: inline-block;
border: 1px solid #000;
width: 20px;
height: 20px;
text-align: center;
background-color: #ccc;
cursor: pointer;
}
</style>
</head>
<body>
<div class="container">
<button onclick="startGame()">开始游戏</button>
</div>
<div class="container">
<div id="grid"></div>
</div>
<script>
var grid = document.getElementById("grid");
var mines = [[1, 3], [2, 2], [3, 4]]; // 雷的位置,示例中有3个雷
var gridSize = 5; // 网格大小为5x5
function startGame() {
grid.innerHTML = ""; // 清空之前的网格
for (var i = 0; i < gridSize; i++) {
for (var j = 0; j < gridSize; j++) {
var cell = document.createElement("div");
cell.classList.add("cell");
// 点击网格时的事件处理函数
cell.onclick = function() {
var x = parseInt(this.getAttribute("data-row"));
var y = parseInt(this.getAttribute("data-col"));
revealCell(x, y);
};
cell.setAttribute("data-row", i);
cell.setAttribute("data-col", j);
grid.appendChild(cell);
}
grid.appendChild(document.createElement("br"));
}
}
function revealCell(row, col) {
var cell = grid.querySelector('[data-row="' + row + '"][data-col="' + col + '"]');
// 点击的格子是雷,游戏结束
if (isMine(row, col)) {
cell.style.backgroundColor = "red";
setTimeout(function() {
alert("游戏结束");
}, 100);
} else {
cell.style.backgroundColor = "white";
}
}
function isMine(row, col) {
for (var i = 0; i < mines.length; i++) {
if (mines[i][0] === row && mines[i][1] === col) {
return true;
}
}
return false;
}
</script>
</body>
</html>
```
这个简单版本的扫雷游戏使用了一个5x5的网格,并通过点击格子的方式来进行游戏。前面有一个"开始游戏"按钮,点击后会生成一个5x5的网格,每个格子都有一个边框,初始颜色为灰色。雷的位置由`mines`数组表示,每个元素是一个包含雷的横坐标和纵坐标的数组。点击格子时,会检查该位置是否为雷,如果是雷则游戏结束,反之则将格子颜色变为白色。
阅读全文
相关推荐
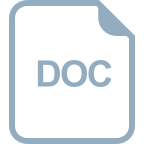
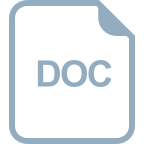
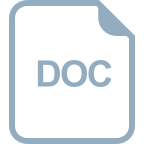
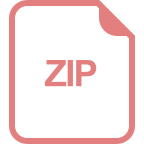
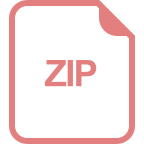
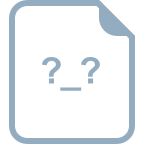
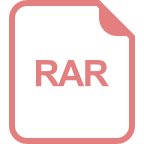
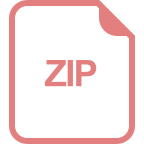
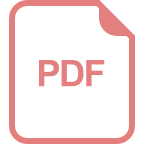
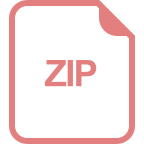








