jupyter notebook读取excel某列数据
时间: 2023-06-16 09:05:10 浏览: 723
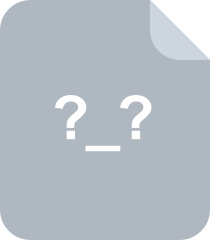
读取excel数据
你可以使用Python中的pandas库来读取Excel文件中的数据。下面是一个示例代码,它读取Excel文件中的第一列数据:
```python
import pandas as pd
# 读取 Excel 文件
df = pd.read_excel('example.xls')
# 获取第一列数据
col_data = df.iloc[:, 0].values.tolist()
# 打印数据
print(col_data)
```
在这个代码中,`read_excel()` 函数用于读取 Excel 文件,`iloc[:, 0]` 用于获取第一列数据,`tolist()` 用于将数据转换为列表形式。你可以根据需要调整列的索引和输出格式。
阅读全文
相关推荐















