stm32串口接收字符串
时间: 2023-09-25 08:16:09 浏览: 174
在STM32上使用串口接收字符串,可以使用中断方式或轮询方式实现。下面是一个使用中断方式接收字符串的示例代码:
```c
#include "stm32f10x.h"
#include <string.h>
#define RX_BUFFER_SIZE 50
// 串口接收缓存区
uint8_t rx_buffer[RX_BUFFER_SIZE];
// 接收计数器
volatile uint8_t rx_counter = 0;
// 接收完成标志
volatile uint8_t rx_complete = 0;
// 串口中断处理函数
void USART1_IRQHandler(void)
{
// 接收数据寄存器非空
if (USART_GetITStatus(USART1, USART_IT_RXNE) == SET)
{
uint8_t data = USART_ReceiveData(USART1);
// 判断是否接收到 '\r' 或 '\n',表示接收完成
if (data == '\r' || data == '\n')
{
rx_buffer[rx_counter] = '\0'; // 添加字符串结束符
rx_complete = 1; // 设置接收完成标志
rx_counter = 0; // 重置接收计数器
}
else
{
rx_buffer[rx_counter++] = data; // 存储接收到的数据
if (rx_counter >= RX_BUFFER_SIZE)
{
rx_counter = 0; // 缓冲区溢出,重置接收计数器
}
}
}
}
int main(void)
{
// 初始化串口1,波特率为115200,8位数据位,1位停止位,无校验位
USART_InitTypeDef USART_InitStructure;
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能串口1接收中断
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_Cmd(USART1, ENABLE); // 使能串口1
while (1)
{
if (rx_complete) // 判断是否接收完成
{
// 处理接收到的字符串
// ...
rx_complete = 0; // 重置接收完成标志
}
}
}
```
在上面的代码中,通过中断方式接收串口数据,并将接收到的数据存储到一个缓存区中,当接收到 '\r' 或 '\n' 字符时,表示字符串接收完成,可以进行后续处理。需要注意的是,在处理接收到的数据时,需要根据实际情况进行解析,避免出现缓冲区溢出或越界等问题。
相关推荐
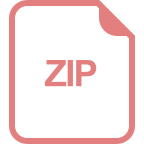
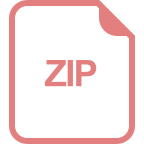
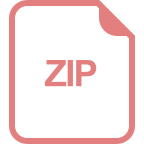
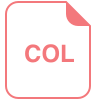
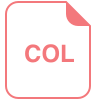
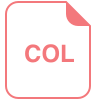
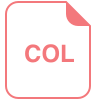
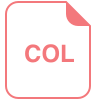









