如何将中缀表达式转换为后缀表达式?
时间: 2024-04-21 11:20:14 浏览: 13
以下是将中缀表达式转换为后缀表达式的方法[^1][^2]:
1. 创建一个空栈和一个空列表,用于存储操作符和最终的后缀表达式。
2. 从左到右遍历中缀表达式的每个字符。
3. 如果当前字符是操作数(数字或变量),则将其添加到后缀表达式列表的末尾。
4. 如果当前字符是左括号"(",则将其压入栈中。
5. 如果当前字符是右括号")",则将栈中的操作符弹出并添加到后缀表达式列表,直到遇到左括号为止。然后将左括号从栈中弹出。
6. 如果当前字符是操作符(+、-、*、/等),则将其与栈顶的操作符进行比较:
- 如果栈为空或栈顶的操作符是左括号"(",则将当前操作符压入栈中。
- 如果当前操作符的优先级大于栈顶的操作符,则将当前操作符压入栈中。
- 如果当前操作符的优先级小于或等于栈顶的操作符,则将栈顶的操作符弹出并添加到后缀表达式列表,然后将当前操作符压入栈中。
7. 当遍历完中缀表达式后,将栈中剩余的操作符依次弹出并添加到后缀表达式列表。
8. 后缀表达式列表即为转换后的后缀表达式。
以下是一个示例代码,将中缀表达式转换为后缀表达式:
```python
def infix_to_postfix(expression):
precedence = {'+': 1, '-': 1, '*': 2, '/': 2}
stack = []
postfix = []
for char in expression:
if char.isalnum():
postfix.append(char)
elif char == '(':
stack.append(char)
elif char == ')':
while stack and stack[-1] != '(':
postfix.append(stack.pop())
stack.pop()
else:
while stack and stack[-1] != '(' and precedence[char] <= precedence.get(stack[-1], 0):
postfix.append(stack.pop())
stack.append(char)
while stack:
postfix.append(stack.pop())
return ''.join(postfix)
infix_expression = "-+6*3-74/82"
postfix_expression = infix_to_postfix(infix_expression)
print(postfix_expression) # 输出:63*74-82/+
```
相关推荐
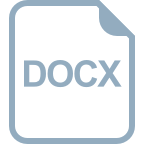
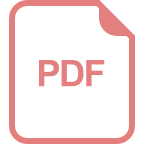
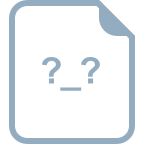














