java定义一个矩形类retangle和三角形triangle类
时间: 2024-11-03 15:22:35 浏览: 3
在Java中,我们可以定义两个类来表示矩形和三角形,每个类都会包含它们特有的属性和方法。这里是一个简单的示例:
```java
// 矩形Rectangle类
public class Rectangle {
private double width; // 宽度
private double height; // 高度
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
// 获取和设置宽度、高度的方法
public double getWidth() { return width; }
public void setWidth(double width) { this.width = width; }
public double getHeight() { return height; }
public void setHeight(double height) { this.height = height; }
// 计算面积的方法
public double getArea() {
return width * height;
}
}
// 三角形Triangle类
public class Triangle {
private double base; // 底边长度
private double height; // 高度
public Triangle(double base, double height) {
this.base = base;
this.height = height;
}
// 获取和设置底边、高度的方法
public double getBase() { return base; }
public void setBase(double base) { this.base = base; }
public double getHeight() { return height; }
public void setHeight(double height) { this.height = height; }
// 计算面积的方法 (海伦公式)
public double getArea() {
double s = (base + height + Math.abs(base - height)) / 2; // 半周长
return Math.sqrt(s * (s - base) * (s - height) * (s - (base + height)));
}
}
```
阅读全文
相关推荐
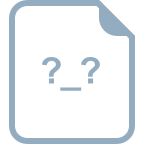
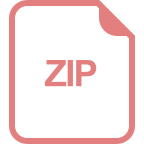
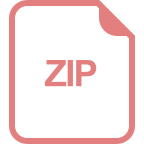















