帮我写一段代码,1)设计一个点的类,属性为坐标x和y,方法有构造方法,set方法,get方法,toString方法等。 (2)设计线段类,属性为两个点,方法有构造方法,set方法,get方法,toString方法以及求两点距离的方法 (3)设计主类,键盘输入任意两个点,求出两点间的距离。
时间: 2023-06-05 09:05:05 浏览: 180
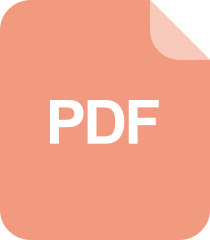
Java 编写Point类,构造方法,计算两个点之间的距离,键盘输入两个点,格式以“,”隔开,如:1,1即为(1,1)点。

好的,我可以帮您写这段代码,以下是代码实现:
点类:
```
public class Point {
private int x;
private int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
@Override
public String toString() {
return "(" + x + "," + y + ")";
}
}
```
线段类:
```
public class Line {
private Point p1;
private Point p2;
public Line(Point p1, Point p2) {
this.p1 = p1;
this.p2 = p2;
}
public void setP1(Point p1) {
this.p1 = p1;
}
public void setP2(Point p2) {
this.p2 = p2;
}
public Point getP1() {
return p1;
}
public Point getP2() {
return p2;
}
public double getLength() {
int deltaX = p2.getX() - p1.getX();
int deltaY = p2.getY() - p1.getY();
return Math.sqrt(deltaX * deltaX + deltaY * deltaY);
}
@Override
public String toString() {
return "Line " + p1.toString() + "-" + p2.toString();
}
}
```
主类:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.println("Please enter the coordinates of point 1:");
int x1 = input.nextInt();
int y1 = input.nextInt();
Point p1 = new Point(x1, y1);
System.out.println("Please enter the coordinates of point 2:");
int x2 = input.nextInt();
int y2 = input.nextInt();
Point p2 = new Point(x2, y2);
Line line = new Line(p1, p2);
System.out.println("The distance between " + p1.toString() + " and " + p2.toString() + " is " + line.getLength());
}
}
```
请注意,上述代码仅供参考,您可以根据需要进行修改。
阅读全文
相关推荐
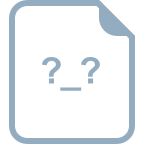
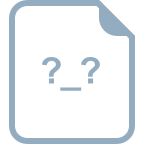
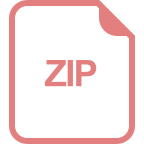
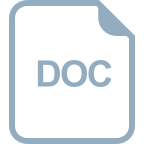
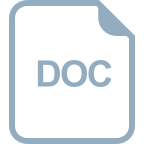
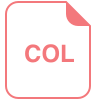
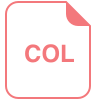









