分析一下下面的代码from scipy.sparse import csgraph import subprocess from scipy import sparse if __name__ == '__main__': print("====================================") row = [2, 3, 3, 2] col = [3, 4, 2, 3] data = [1, 2, 3, 10] c = sparse.coo_matrix((data, (row, col)), shape=(5, 6)) print(c.col, c.row, c.data) print(c.toarray()) print("====================================") # Find the shortest path # Define the graph in the DOT language code = """ digraph graph1{ rankdir=LR; size="8,5" node [shape = circle]; A -> B [ label = "10" ]; B -> C [ label = "5" ]; A -> C [ label = "3" ]; C -> D [ label = "7" ]; D -> A [ label = "4" ]; D -> C [ label = "6" ]; } """ # Define the command and its arguments to run Graphviz dot_args = ["dot", "-T", "svg"] # Run Graphviz and pass the DOT code as input p = subprocess.Popen(dot_args, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE) stdout, stderr = p.communicate(code.encode('utf-8')) # Save the SVG image to a file with open("output.svg", "w") as f: f.write(stdout.decode("utf-8")) w = sparse.dok_matrix((4,4)) edges = [(0, 1, 10), (1, 2, 5), (0, 2, 3), (2, 3, 7), (3, 0, 4), (3, 2, 6)] for i, j, v in edges: w[i, j] = v d, p = csgraph.dijkstra(csgraph=w, directed=True, indices=0, return_predecessors=True) print(d) print(p)
时间: 2023-08-13 12:07:44 浏览: 209
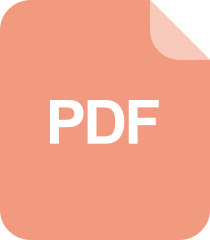
Python错题本:from scipy.misc import imread 报错cannot import name imread 的解决方案
这段代码主要是使用Python中的一些科学计算库和系统命令来实现以下两个功能:
1. 利用scipy库构建一个稀疏矩阵,并对其进行一些基本操作,比如输出矩阵的列、行、非零元素等信息,以及将其转换为稠密矩阵。
2. 利用Graphviz工具绘制一个有向图,并利用scipy库中的函数求解该有向图的最短路径和路径前驱。
具体分析如下:
代码中首先引入了需要用到的库:scipy.sparse.csgraph、subprocess和scipy.sparse。其中,scipy.sparse.csgraph库提供了计算稀疏矩阵最短路径的函数,subprocess库提供了运行系统命令的函数,而scipy.sparse库则提供了构建稀疏矩阵的函数。
接下来的if __name__ == '__main__':语句块是Python中经常使用的用法,用于标识当前脚本是否为主程序入口。
在if语句块中,首先创建了一个COO格式的稀疏矩阵c,并对其进行了一些基本操作,比如输出矩阵的列、行、非零元素等信息,以及将其转换为稠密矩阵。
然后,定义了一个DOT格式的有向图代码,并利用subprocess库中的Popen函数运行Graphviz工具,并将DOT代码作为输入传递给它,以生成一个SVG格式的图像文件。
最后,利用scipy.sparse库中的函数构建了一个四个节点的有向图,并利用scipy.sparse.csgraph库中的dijkstra函数求解该图的最短路径和路径前驱,并输出结果。
阅读全文
相关推荐
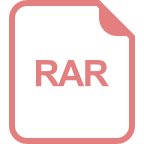















