利用python编写一个提取图片中的矩形的代码
时间: 2023-03-29 14:04:10 浏览: 89
你好,我是 CSDN 开发的 C 知道。关于你的问题,我可以回答。利用 Python 可以使用 OpenCV 库来提取图片中的矩形。具体的代码实现可以参考以下步骤:
1. 导入 OpenCV 库
import cv2
2. 读取图片
img = cv2.imread('image.jpg')
3. 将图片转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
4. 进行边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
5. 进行轮廓检测
contours, hierarchy = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
6. 遍历轮廓,找到矩形
for cnt in contours:
approx = cv2.approxPolyDP(cnt, .01*cv2.arcLength(cnt, True), True)
if len(approx) == 4:
cv2.drawContours(img, [approx], , (, 255, ), 2)
7. 显示结果
cv2.imshow('image', img)
cv2.waitKey()
cv2.destroyAllWindows()
以上是提取图片中矩形的代码实现。希望对你有所帮助。
相关问题
用python编写代码,实现基于Hough变换的“矩形矫正”,和瓶子盖轮廓提取
在Python中,你可以使用OpenCV库来实现基于Hough变换的矩形矫正和瓶子盖轮廓提取。Hough变换是一种用于检测图像中的直线、圆或其他形状特征的经典计算机视觉技术。
首先,确保已经安装了`opencv-python`库,如果没有可以使用pip进行安装:
```bash
pip install opencv-python
```
下面是一个简单的例子,展示如何进行这两个步骤:
**1. 矩形矫正:**
假设我们有一个倾斜的矩形图片,我们可以先找到图片中的角点(通常通过边缘检测和非极大值抑制),然后用Hough变换找出最接近矩形的角度,并对图片进行旋转矫正。
```python
import cv2
import numpy as np
def rotate_image(image, angle):
# 获取图像中心点
height, width = image.shape[:2]
center = (width // 2, height // 2)
# 计算旋转矩阵
rotation_matrix = cv2.getRotationMatrix2D(center, angle, scale=1)
# 应用旋转并返回
rotated_image = cv2.warpAffine(image, rotation_matrix, (width, height))
return rotated_image
# 加载图像并进行灰度处理
image = cv2.imread('input_image.jpg', cv2.IMREAD_GRAYSCALE)
edges = cv2.Canny(image, threshold1=50, threshold2=150) # 边缘检测
# 找到角点
vertices = cv2.goodFeaturesToTrack(edges, minDistance=5, maxCorners=4, qualityLevel=0.01, minRepeatability=3)
corners = np.int0(cv2.cornerSubPix(image, vertices, (5, 5), (-1, -1), criteria))
# 使用Hough变换找到可能的矩形角度
lines = cv2.HoughLinesP(edges, rho=1, theta=np.pi / 180, threshold=100, lines=np.array([]), minLineLength=50, maxLineGap=10)
if lines is not None:
angles = [line[1][1] for line in lines]
angle = np.mean(angles)
rotated_image = rotate_image(image, angle * 180 / np.pi) # 根据角度旋转图像
cv2.imwrite('rotated_image.jpg', rotated_image)
```
**2. 瓶子盖轮廓提取:**
对于瓶子盖轮廓,你可能会用霍夫环检测来识别圆形,然后再结合膨胀操作来寻找相对完整的轮廓。
```python
# 对矫正后的图像进行二值化
_, binary = cv2.threshold(rotated_image, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
# 霍夫环检测找圆
circles = cv2.HoughCircles(binary, cv2.HOUGH_GRADIENT, dp=1, minDist=50, param1=50, param2=30, minRadius=10, maxRadius=50)
if circles is not None:
circles = np.round(circles[0, :]).astype("int")
for (x, y, r) in circles:
cv2.circle(rotated_image, (x, y), r, (0, 255, 0), 2) # 绘制轮廓
cropped_circle = rotated_image[y-r:y+r+1, x-x:x+x+1] # 提取轮廓区域
cv2.imshow('Bottle Cap Outline', rotated_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请用Python编写代码,实现基于Hough变换的“矩形矫正”,和瓶子盖轮廓提取。
在Python中,我们可以使用OpenCV库来实现基于霍夫变换的矩形校正以及图像处理中的瓶子盖轮廓提取。首先,我们需要导入必要的模块:
```python
import cv2
import numpy as np
# 加载图片
img = cv2.imread('bottle_cap.jpg', cv2.IMREAD_GRAYSCALE)
```
**矩形矫正:**
霍夫变换通常用于直线检测,但对于矩形,我们可以通过对角线来寻找接近正方形的四边形。以下是一个简单的步骤:
```python
def detect_rectangle(hough_lines):
# 提取对角线
diagonal_lines = [line for line in hough_lines if line[0][1] == line[1][1]]
# 计算对角线交点
x, y = zip(*diagonal_lines)
x, y = np.mean(x), np.mean(y)
# 使用矩形中心作为参考点,调整边界框
height, width = img.shape
top_left = (int(x - width / 2), int(y - height / 2))
bottom_right = (int(x + width / 2), int(y + height / 2))
return top_left, bottom_right
# 使用霍夫变换找边缘并提取对角线
lines = cv2.HoughLinesP(img, rho=1, theta=np.pi / 180, threshold=50, minLineLength=100, maxLineGap=10)
top_left, bottom_right = detect_rectangle(lines)
# 绘制矫正后的矩形区域
corrected_img = cv2.rectangle(img, top_left, bottom_right, (0, 255, 0), 2)
```
**瓶子盖轮廓提取:**
对于轮廓提取,可以使用`cv2.findContours()`函数:
```python
contours, hierarchy = cv2.findContours(img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 筛选可能是瓶盖的轮廓
min_area = 1000 # 可能需要根据实际情况调整
good_contours = [cnt for cnt in contours if cv2.contourArea(cnt) > min_area]
# 对每个找到的轮廓进行进一步处理(比如填充、边界等)
for contour in good_contours:
rotated_contour = rotate_bound(contour, angle) # 根据实际需求添加旋转计算
bottle_cap = draw_contour(rotated_contour, corrected_img) # 根据需要绘制轮廓到新图上
# 函数示例(未提供完整代码,仅作说明)
def rotate_bound(image, angle):
# ... 实现旋转功能 ...
def draw_contour(contour, img):
# ... 实现轮廓描边或填充功能 ...
```
这只是一个基本的框架,具体的细节可能需要根据目标图像的特性和需求进行优化。
阅读全文
相关推荐
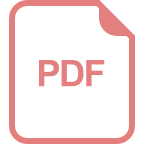
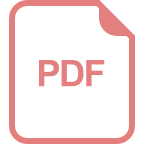
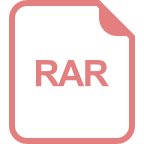
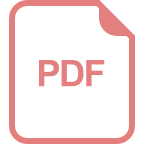
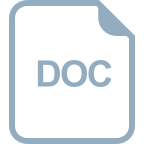
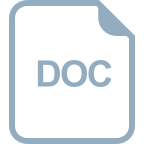
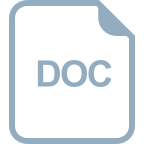
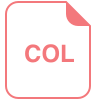
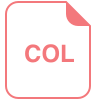
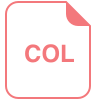



