def on_message(client, userdata, msg): msg = json.loads(msg.payload) print(msg) daction = msg['items']['action']['value'] if daction[2] == '36': SSR.start_action_thread() SSR.runAction(action) SSR.change_action_value('11', 1) else: SSR.runAction(daction)怎么取action值
时间: 2024-02-07 11:03:23 浏览: 60
在这段代码中,`msg`是一个JSON格式的消息,需要使用`json.loads()`方法将其转换为Python字典对象。然后,可以使用`msg['items']['action']['value']`来获取`action`值。
所以,要取出`action`的值可以使用如下代码:
```
msg = json.loads(msg.payload)
action = msg['items']['action']['value']
```
这将把`action`的值存储在`action`变量中,可以在后续的代码中使用。在这段代码中,`daction`变量和`action`变量是相同的,因为它们都存储了相同的值。所以,可以将这段代码修改为:
```
msg = json.loads(msg.payload)
action = msg['items']['action']['value']
if action[2] == '36':
SSR.start_action_thread()
SSR.runAction(action)
SSR.change_action_value('11', 1)
else:
SSR.runAction(action)
```
这将根据`action`值的第三个字符是否为'36'来执行不同的操作。
相关问题
def on_message(client, userdata, msg): msg=json.loads(msg.payload) print(msg) action=msg['items']['action']['value'] if action == '0': SSR.start_action_thread() SSR.runAction(action) SSR.change_action_value('11',1) else: SSR.runAction(action)语法错误
这段代码中的语法错误是缩进错误。请参考下面的代码修复此问题:
```
def on_message(client, userdata, msg):
msg = json.loads(msg.payload)
print(msg)
action = msg['items']['action']['value']
if action == '0':
SSR.start_action_thread()
SSR.runAction(action)
SSR.change_action_value('11', 1)
else:
SSR.runAction(action)
```
这是一个 MQTT 消息回调函数,当接收到消息时会被调用。函数中首先将消息的载荷解析为 JSON 格式,并打印出来。然后从消息中提取出 `action` 的值,根据这个值来执行不同的动作。如果 `action` 的值是字符串 `'0'`,则启动一个新线程执行动作,运行动作,修改动作的值为 `'11'`,否则只是运行动作。
modify the program so that this “b” no longer appears:import paho.mqtt.client as mqtt def on_connect(client,userdata,flags,rc): print("Connected with result code"+str(rc)) client.subscribe("hello/#") def on_message(client,userdata,msg): print(msg.topic+" "+str(msg.payload)) client=mqtt.Client() client.on_connect=on_connect client.on_message=on_message print("Connecting") client.connect("localhost",1883,60) client.loop_forever()
The "b" in the output can be removed by decoding the byte payload into a string. Here's the modified code:
```python
import paho.mqtt.client as mqtt
def on_connect(client, userdata, flags, rc):
print("Connected with result code " + str(rc))
client.subscribe("hello/#")
def on_message(client, userdata, msg):
print(msg.topic + " " + msg.payload.decode('utf-8')) # Decode the byte payload to string
client = mqtt.Client()
client.on_connect = on_connect
client.on_message = on_message
print("Connecting")
client.connect("localhost", 1883, 60)
client.loop_forever()
```
By using the `decode('utf-8')` method on the `msg.payload`, we convert the byte payload to a string using the UTF-8 encoding. This ensures that the output no longer includes the "b" indicating a byte literal.
阅读全文
相关推荐
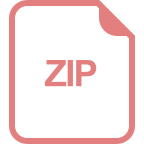
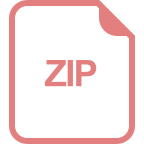
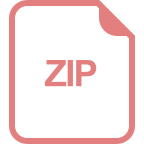













