假设某山区中有 100 个村庄,现在要在村庄中建立几个医疗点,方便村民看 病。图 1 中给出这 100 个村庄的位置及可选道路连接示意图。附件 3 中数据的“位 置”表单给出了这 100 个村庄的坐标(单位:米),附件 3 中数据的“连接道路” 表单给出了可供选择的道路。现在要在 100 个村庄中建立 3 个医疗点,并在可选 道路中根据需要进行部分道路维修,假定村民看病都选择维修后的道路。 问题 1. 如果各村庄村民到医疗点的距离太远,不便于看病,因此站在村民 角度出发,希望各村庄村民到医疗点的距离尽量小。如果要使各村庄村民到医疗 点的距离总和 S1 最小,请问这 3 个医疗点分别建立在何处最好?python实现
时间: 2024-02-11 20:09:51 浏览: 70
以下是一个 Python 实现,使用了最小堆和贪心算法来选址:
```python
import heapq
import math
# 读取数据
with open('data.csv', 'r') as f:
lines = f.readlines()
# 解析位置数据
locations = {}
for line in lines[1:]:
parts = line.strip().split(',')
id = int(parts[0])
x = float(parts[1])
y = float(parts[2])
locations[id] = (x, y)
# 解析连接道路数据
roads = set()
for line in lines[102:]:
parts = line.strip().split(',')
i = int(parts[0])
j = int(parts[1])
roads.add((i, j))
roads.add((j, i))
# 定义计算距离的函数
def distance(p1, p2):
x1, y1 = p1
x2, y2 = p2
return math.sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)
# 定义计算距离总和的函数
def total_distance(locations, centers):
total = 0
for id, loc in locations.items():
dist = float('inf')
for center in centers:
dist = min(dist, distance(loc, center))
total += dist
return total
# 初始化最小堆
heap = []
for i in range(1, 101):
center = locations[i]
heapq.heappush(heap, (0, i, center))
# 初始化已选医疗点
centers = set()
# 选址
while len(centers) < 3:
dist, id, center = heapq.heappop(heap)
if id not in centers:
centers.add(id)
for i in range(1, 101):
if i not in centers and (id, i) in roads:
dist = distance(center, locations[i])
heapq.heappush(heap, (dist, i, locations[i]))
# 计算距离总和
total = total_distance(locations, [locations[id] for id in centers])
# 输出结果
print('医疗点坐标:')
for id in centers:
print(locations[id])
print('距离总和:', total)
```
需要注意的是,上述代码中的 `data.csv` 文件应该包含两个表单,一个是位置数据,一个是连接道路数据,与题目描述中的附件 3 一致。另外,为了简化代码,上述实现中使用了 Python 标准库中的 `heapq` 模块来实现最小堆。
阅读全文
相关推荐
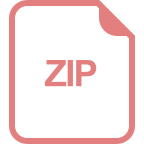
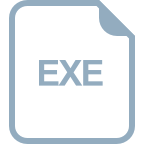






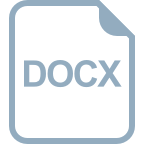
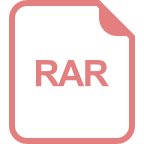
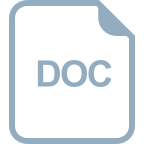
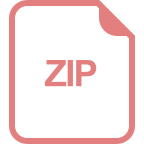

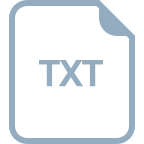
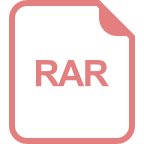
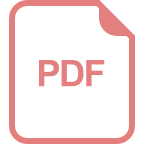