数据结构编写程序实现哈夫曼树构造过程。 代码:
时间: 2024-05-14 13:18:25 浏览: 135
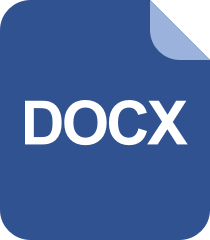
头歌数据结构构建哈夫曼树及编码

以下是使用C++语言实现哈夫曼树构造的代码:
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 定义哈夫曼树节点结构体
struct HuffNode {
char data; // 字符
int weight; // 权重
int parent; // 父节点下标
int lchild; // 左孩子下标
int rchild; // 右孩子下标
};
// 定义比较函数
struct cmp {
bool operator()(const HuffNode& a, const HuffNode& b) const {
return a.weight > b.weight;
}
};
// 构造哈夫曼树
HuffNode* buildHuffmanTree(vector<char>& chars, vector<int>& weights) {
int n = chars.size();
priority_queue<HuffNode, vector<HuffNode>, cmp> q; // 定义小根堆
HuffNode* nodes = new HuffNode[2 * n - 1]; // 定义哈夫曼树节点数组
// 初始化叶子节点
for (int i = 0; i < n; i++) {
nodes[i].data = chars[i];
nodes[i].weight = weights[i];
nodes[i].parent = -1;
nodes[i].lchild = -1;
nodes[i].rchild = -1;
q.push(nodes[i]); // 将叶子节点插入小根堆中
}
int k = n; // 记录节点数组中下一个可用下标
for (int i = 0; i < n - 1; i++) {
HuffNode node1 = q.top(); // 取出小根堆中最小的两个节点
q.pop();
HuffNode node2 = q.top();
q.pop();
// 创建一个新节点,作为node1和node2的父节点
nodes[k].data = '#';
nodes[k].weight = node1.weight + node2.weight;
nodes[k].parent = -1;
nodes[k].lchild = node1.weight <= node2.weight ? node1.parent : node2.parent;
nodes[k].rchild = node1.weight <= node2.weight ? node2.parent : node1.parent;
node1.parent = k;
node2.parent = k;
q.push(nodes[k]); // 将新节点插入小根堆中
k++;
}
return nodes;
}
int main() {
vector<char> chars = {'A', 'B', 'C', 'D', 'E', 'F'};
vector<int> weights = {5, 1, 6, 3, 8, 4};
HuffNode* nodes = buildHuffmanTree(chars, weights);
// 输出哈夫曼树
for (int i = 0; i < 2 * chars.size() - 1; i++) {
cout << "Node " << i << ": ";
cout << "data=" << nodes[i].data << ", ";
cout << "weight=" << nodes[i].weight << ", ";
cout << "parent=" << nodes[i].parent << ", ";
cout << "lchild=" << nodes[i].lchild << ", ";
cout << "rchild=" << nodes[i].rchild << endl;
}
delete[] nodes; // 释放内存
return 0;
}
```
以上代码中,使用了一个小根堆来存储所有的叶子节点,并且每次取出堆中最小的两个节点,通过合并创建一个新节点,并将新节点插入堆中。最后,堆中仅剩一个节点,即为根节点。其中,节点结构体中包含了字符、权重、父节点下标、左孩子下标和右孩子下标等信息。
阅读全文
相关推荐
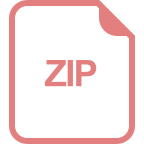
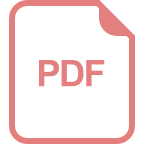















