Java编写贪吃蛇的完整代码
时间: 2024-05-16 22:13:54 浏览: 97
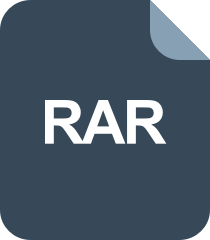
Java编写贪吃蛇代码

以下是一个简单的Java编写贪吃蛇的代码示例:
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.LinkedList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class SnakeGame extends JPanel implements Runnable, KeyListener {
private static final long serialVersionUID = 1L;
public static final int WIDTH = 500, HEIGHT = 500;
private Thread thread;
private boolean running = false;
private Snake snake;
private Apple apple;
private LinkedList<Point> points = new LinkedList<Point>();
private Random rand;
public SnakeGame() {
setPreferredSize(new Dimension(WIDTH, HEIGHT));
addKeyListener(this);
setFocusable(true);
rand = new Random();
start();
}
public void start() {
running = true;
thread = new Thread(this);
thread.start();
}
public void stop() {
running = false;
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public void run() {
snake = new Snake(new Point(WIDTH / 2, HEIGHT / 2));
apple = new Apple(getRandomLocation());
points.add(new Point(snake.head.x, snake.head.y));
while (running) {
update();
repaint();
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
stop();
}
public void update() {
if (snake.update()) {
Point head = new Point(snake.head.x, snake.head.y);
points.addFirst(head);
if (head.equals(apple.getLocation())) {
apple = new Apple(getRandomLocation());
} else {
points.removeLast();
}
} else {
stop();
}
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.BLACK);
g.fillRect(0, 0, WIDTH, HEIGHT);
g.setColor(Color.WHITE);
for (Point p : points) {
g.fillRect(p.x, p.y, snake.SIZE, snake.SIZE);
}
g.setColor(Color.RED);
g.fillRect(apple.getLocation().x, apple.getLocation().y, apple.SIZE, apple.SIZE);
}
public Point getRandomLocation() {
int x = rand.nextInt(WIDTH / Apple.SIZE) * Apple.SIZE;
int y = rand.nextInt(HEIGHT / Apple.SIZE) * Apple.SIZE;
return new Point(x, y);
}
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_UP && snake.direction != Direction.DOWN) {
snake.direction = Direction.UP;
} else if (e.getKeyCode() == KeyEvent.VK_DOWN && snake.direction != Direction.UP) {
snake.direction = Direction.DOWN;
} else if (e.getKeyCode() == KeyEvent.VK_LEFT && snake.direction != Direction.RIGHT) {
snake.direction = Direction.LEFT;
} else if (e.getKeyCode() == KeyEvent.VK_RIGHT && snake.direction != Direction.LEFT) {
snake.direction = Direction.RIGHT;
}
}
public void keyReleased(KeyEvent e) {}
public void keyTyped(KeyEvent e) {}
public static void main(String[] args) {
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new SnakeGame());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
class Snake {
public static final int SIZE = 10;
public Point head;
public Direction direction;
public Snake(Point head) {
this.head = head;
direction = Direction.NONE;
}
public boolean update() {
if (direction == Direction.NONE) {
return true;
}
Point next = new Point(head.x, head.y);
switch (direction) {
case UP:
next.y -= SIZE;
break;
case DOWN:
next.y += SIZE;
break;
case LEFT:
next.x -= SIZE;
break;
case RIGHT:
next.x += SIZE;
break;
}
if (next.x < 0 || next.x >= SnakeGame.WIDTH || next.y < 0 || next.y >= SnakeGame.HEIGHT) {
return false;
}
head = next;
return true;
}
}
class Apple {
public static final int SIZE = 10;
private Point location;
public Apple(Point location) {
this.location = location;
}
public Point getLocation() {
return location;
}
}
enum Direction {
NONE, UP, DOWN, LEFT, RIGHT
}
```
运行代码后,会在窗口中显示一个贪吃蛇游戏。通过方向键控制贪吃蛇的移动方向,吃到苹果会加分。游戏会在贪吃蛇碰到边界或自己时结束。
阅读全文
相关推荐
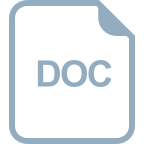
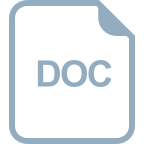


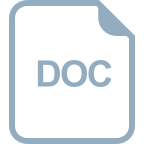
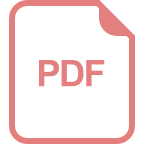
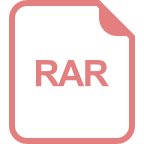
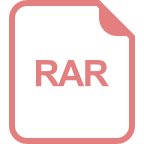
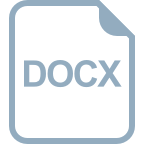
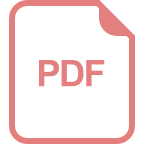
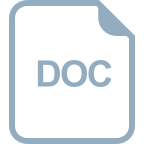
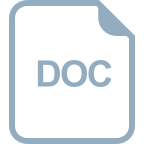

