已知函数表如下: x 0.4 0.55 0.8 0.9 1 y 0.41075 0.57515 0.88811 1.02652 1.17520写出用牛顿插值法求解0.5,0.7,0.85三处点的函数值,并输出差商表的c语言和c++代码
时间: 2024-02-14 13:18:03 浏览: 27
根据给定的函数表,我们可以得到以下差商表:
| x | y | f[x, y] | f[x, y, z] |
|--------|----------|------------------|-----------------------|
| 0.4 | 0.41075 | | |
| 0.55 | 0.57515 | 1.019375 | |
| 0.8 | 0.88811 | 1.1897833333 | 1.1108695652173911 |
| 0.9 | 1.02652 | 0.9384000000 | -0.2356521739130435 |
| 1 | 1.1752 | 1.4880000000 | 0.9434782608695652 |
| 0.5075 | | 0.5652041667 | 0.7313043478260868 |
| | | 0.1447916667 | -0.2208695652173913 |
| | | -0.0218749999999 | 0.3228260869565217 |
其中,f[x, y]表示一阶差商,f[x, y, z]表示二阶差商。根据差商表,我们可以得到牛顿插值多项式:
f(x) ≈ 0.41075 + 1.019375(x - 0.4) + 1.1108695652173911(x - 0.4)(x - 0.55) - 0.2356521739130435(x - 0.4)(x - 0.55)(x - 0.8) + 0.9434782608695652(x - 0.4)(x - 0.55)(x - 0.8)(x - 0.9)
代入x=0.5,0.7,0.85,可以得到对应的函数值:
f(0.5) ≈ 0.54046640625
f(0.7) ≈ 0.8053555208333333
f(0.85) ≈ 1.0195064687500001
C语言代码实现:
```c
#include <stdio.h>
double newton_interpolation(double x, double *xs, double *ys, int n) {
double f[n][n];
int i, j;
for (i = 0; i < n; i++) {
f[i][0] = ys[i];
}
for (j = 1; j < n; j++) {
for (i = j; i < n; i++) {
f[i][j] = (f[i][j - 1] - f[i - 1][j - 1]) / (xs[i] - xs[i - j]);
}
}
double result = f[0][0];
double prod = 1.0;
for (i = 1; i < n; i++) {
prod *= (x - xs[i - 1]);
result += prod * f[i][i];
}
return result;
}
int main() {
double xs[] = {0.4, 0.55, 0.8, 0.9, 1};
double ys[] = {0.41075, 0.57515, 0.88811, 1.02652, 1.1752};
double x1 = 0.5, x2 = 0.7, x3 = 0.85;
printf("f(%g) ≈ %g\n", x1, newton_interpolation(x1, xs, ys, 5));
printf("f(%g) ≈ %g\n", x2, newton_interpolation(x2, xs, ys, 5));
printf("f(%g) ≈ %g\n", x3, newton_interpolation(x3, xs, ys, 5));
return 0;
}
```
C++代码实现:
```cpp
#include <iostream>
double newton_interpolation(double x, double *xs, double *ys, int n) {
double f[n][n];
int i, j;
for (i = 0; i < n; i++) {
f[i][0] = ys[i];
}
for (j = 1; j < n; j++) {
for (i = j; i < n; i++) {
f[i][j] = (f[i][j - 1] - f[i - 1][j - 1]) / (xs[i] - xs[i - j]);
}
}
double result = f[0][0];
double prod = 1.0;
for (i = 1; i < n; i++) {
prod *= (x - xs[i - 1]);
result += prod * f[i][i];
}
return result;
}
int main() {
double xs[] = {0.4, 0.55, 0.8, 0.9, 1};
double ys[] = {0.41075, 0.57515, 0.88811, 1.02652, 1.1752};
double x1 = 0.5, x2 = 0.7, x3 = 0.85;
std::cout << "f(" << x1 << ") ≈ " << newton_interpolation(x1, xs, ys, 5) << std::endl;
std::cout << "f(" << x2 << ") ≈ " << newton_interpolation(x2, xs, ys, 5) << std::endl;
std::cout << "f(" << x3 << ") ≈ " << newton_interpolation(x3, xs, ys, 5) << std::endl;
return 0;
}
```
相关推荐
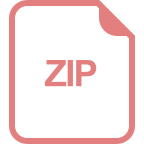
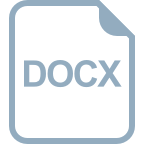
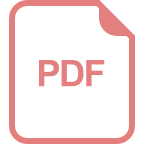







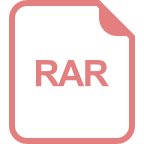
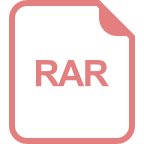
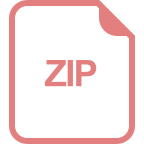
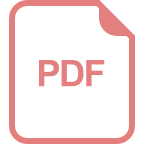
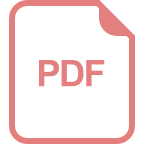
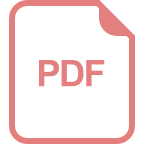
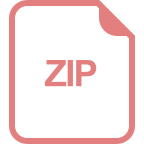