用c++实现扫描线种子填充
时间: 2023-10-13 14:06:23 浏览: 81
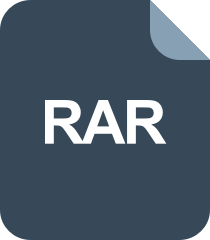
c++实现扫描线填充算法

以下是一个使用C++实现扫描线种子填充算法的示例代码:
```cpp
#include <iostream>
#include <queue>
using namespace std;
// 定义像素点结构体
struct Point {
int x, y;
};
// 定义颜色结构体
struct Color {
int r, g, b;
};
// 定义边界颜色
const Color boundaryColor = {255, 255, 255};
// 定义填充颜色
const Color fillColor = {0, 255, 0};
// 获取像素点的颜色
Color getPixelColor(Point p) {
// 假设这里是获取像素点颜色的函数,根据实际情况进行实现
// 返回颜色值的结构体Color,包含r、g、b三个分量
// 这里只是简单地返回填充颜色
return fillColor;
}
// 设置像素点的颜色
void setPixelColor(Point p, Color c) {
// 假设这里是设置像素点颜色的函数,根据实际情况进行实现
// 输入参数为点的坐标p和颜色值的结构体c,设置点p的颜色为c
}
// 扫描线种子填充算法
void scanlineSeedFill(Point seed) {
queue<Point> pointQueue;
pointQueue.push(seed);
while (!pointQueue.empty()) {
Point current = pointQueue.front();
pointQueue.pop();
if (getPixelColor(current) == boundaryColor) {
continue;
}
setPixelColor(current, fillColor);
Point left = {current.x - 1, current.y};
Point right = {current.x + 1, current.y};
// 向左填充
while (getPixelColor(left) != boundaryColor && getPixelColor(left) != fillColor) {
setPixelColor(left, fillColor);
left.x--;
}
// 向右填充
while (getPixelColor(right) != boundaryColor && getPixelColor(right) != fillColor) {
setPixelColor(right, fillColor);
right.x++;
}
// 检查上一行和下一行
Point up = {current.x, current.y - 1};
Point down = {current.x, current.y + 1};
if (getPixelColor(up) != boundaryColor && getPixelColor(up) != fillColor) {
pointQueue.push(up);
}
if (getPixelColor(down) != boundaryColor && getPixelColor(down) != fillColor) {
pointQueue.push(down);
}
}
}
int main() {
// 假设有一个图像,包含多个像素点
// 这里只是简单的示例,假设图像大小为10x10
const int width = 10;
const int height = 10;
// 初始化图像像素点颜色
Color image[width][height];
// 假设所有像素点初始颜色为边界颜色
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
image[i][j] = boundaryColor;
}
}
// 假设填充区域的种子点为(5, 5)
Point seed = {5, 5};
// 调用扫描线种子填充算法
scanlineSeedFill(seed);
// 输出填充后的图像
for (int i = 0; i < width; i++) {
for (int j = 0; j < height; j++) {
cout << "(" << image[i][j].r << "," << image[i][j].g << "," << image[i][j].b << ") ";
}
cout << endl;
}
return 0;
}
```
以上代码演示了一个简单的扫描线种子填充算法的实现,其中使用了一个队列来存储需要填充的点。通过不断出队并检查该点周围的像素,实现了向上、向下、向左、向右的填充操作。在实际应用中,你可能需要根据具体情况进行一些优化和适配。
阅读全文
相关推荐


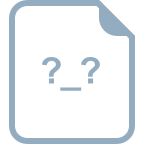
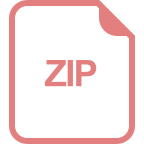
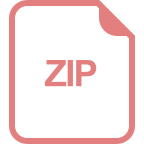
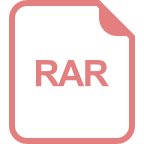
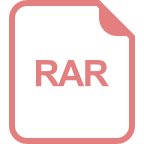
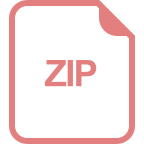





