C++扫描线种子填充算法怎么实现
时间: 2023-07-15 18:15:44 浏览: 142
扫描线种子填充算法是一种用于图形填充的算法,其基本思想是从种子点开始,沿着扫描线进行像素填充,直到遇到边界为止。以下是C++实现该算法的基本步骤:
1. 定义一个像素点结构体,用于存储像素的坐标信息和颜色信息。
```cpp
struct Pixel {
int x;
int y;
int color;
};
```
2. 定义一个扫描线结构体,用于存储扫描线的起始和终止位置。
```cpp
struct ScanLine {
int y;
int left;
int right;
};
```
3. 定义一个函数用于判断像素点是否在边界内部。
```cpp
bool insideBoundary(int x, int y) {
// 判断像素点是否在边界内部
// 如果在内部返回 true,否则返回 false
}
```
4. 定义一个函数用于判断像素点是否为种子点。
```cpp
bool isSeedPoint(int x, int y) {
// 判断像素点是否为种子点
// 如果是返回 true,否则返回 false
}
```
5. 定义一个函数用于填充扫描线上的像素点。
```cpp
void fillScanLine(int y, int left, int right, int color) {
for (int x = left; x <= right; x++) {
// 填充像素点
}
}
```
6. 定义一个函数用于进行扫描线种子填充。
```cpp
void scanLineSeedFill(int x, int y, int color) {
if (!insideBoundary(x, y) || isSeedPoint(x, y)) {
return;
}
// 初始化扫描线
std::vector<ScanLine> scanLines;
scanLines.push_back({y, x, x});
// 循环处理扫描线,直到所有像素点都填充完成
while (!scanLines.empty()) {
// 取出当前扫描线
ScanLine scanLine = scanLines.back();
scanLines.pop_back();
// 填充当前扫描线上的像素点
fillScanLine(scanLine.y, scanLine.left, scanLine.right, color);
// 处理下一扫描线
for (int i = scanLine.left; i <= scanLine.right; i++) {
// 处理当前像素点的上方像素点
if (insideBoundary(i, scanLine.y - 1) && !isSeedPoint(i, scanLine.y - 1)) {
int j = i;
while (insideBoundary(j, scanLine.y - 1) && !isSeedPoint(j, scanLine.y - 1)) {
j--;
}
scanLines.push_back({scanLine.y - 1, j + 1, i - 1});
}
// 处理当前像素点的下方像素点
if (insideBoundary(i, scanLine.y + 1) && !isSeedPoint(i, scanLine.y + 1)) {
int j = i;
while (insideBoundary(j, scanLine.y + 1) && !isSeedPoint(j, scanLine.y + 1)) {
j--;
}
scanLines.push_back({scanLine.y + 1, j + 1, i - 1});
}
}
}
}
```
以上是C++实现扫描线种子填充算法的基本步骤,可以根据具体需求进行调整和优化。
阅读全文
相关推荐
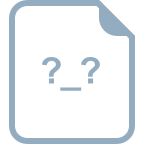
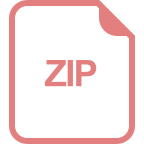




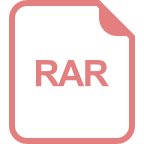
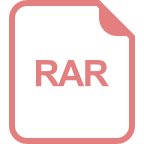
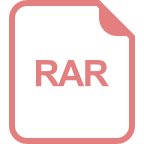




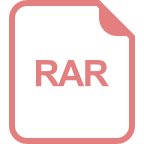