python opencv opencv perspectiveTransform
时间: 2024-04-08 16:28:08 浏览: 120
以下是使用Python OpenCV中的`cv2.getPerspectiveTransform`函数进行透视变换的示例代码[^2]:
```python
import cv2
import numpy as np
# 定义原始图像的四个顶点坐标
pts1 = np.float32([[56, 65], [368, 52], [28, 387], [389, 390]])
# 定义目标图像的四个顶点坐标
pts2 = np.float32([[0, 0], [300, 0], [0, 300], [300, 300]])
# 读取原始图像
img = cv2.imread('input.jpg')
# 进行透视变换
M = cv2.getPerspectiveTransform(pts1, pts2)
dst = cv2.warpPerspective(img, M, (300, 300))
# 显示结果图像
cv2.imshow('Input', img)
cv2.imshow('Output', dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先定义了原始图像的四个顶点坐标`pts1`和目标图像的四个顶点坐标`pts2`。然后使用`cv2.getPerspectiveTransform`函数计算透视变换矩阵`M`。最后,使用`cv2.warpPerspective`函数将原始图像进行透视变换得到目标图像`dst`。最后,通过`cv2.imshow`函数显示原始图像和目标图像。
相关问题
python opencv 图像sift配准拼接
1. 导入库
```
import cv2
import numpy as np
```
2. 读取图片
```
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
```
3. 提取关键点和特征向量
```
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1,None)
kp2, des2 = sift.detectAndCompute(img2,None)
```
4. 匹配特征点
```
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1,des2,k=2)
```
5. 筛选匹配点
```
good = []
for m,n in matches:
if m.distance < 0.75*n.distance:
good.append(m)
```
6. 计算变换矩阵
```
src_pts = np.float32([ kp1[m.queryIdx].pt for m in good ]).reshape(-1,1,2)
dst_pts = np.float32([ kp2[m.trainIdx].pt for m in good ]).reshape(-1,1,2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC,5.0)
```
7. 图像拼接
```
h,w = img1.shape[:2]
pts = np.float32([ [0,0],[0,h-1],[w-1,h-1],[w-1,0] ]).reshape(-1,1,2)
dst = cv2.perspectiveTransform(pts,M)
dst = np.int32(dst)
offset = np.array([w,0])
dst += offset
result_img = cv2.polylines(img2,[dst],True,(255,0,0),3, cv2.LINE_AA)
result_img[offset[1]:h+offset[1],:w,:] = img1
```
完整代码:
```
import cv2
import numpy as np
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1,None)
kp2, des2 = sift.detectAndCompute(img2,None)
bf = cv2.BFMatcher()
matches = bf.knnMatch(des1,des2,k=2)
good = []
for m,n in matches:
if m.distance < 0.75*n.distance:
good.append(m)
src_pts = np.float32([ kp1[m.queryIdx].pt for m in good ]).reshape(-1,1,2)
dst_pts = np.float32([ kp2[m.trainIdx].pt for m in good ]).reshape(-1,1,2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC,5.0)
h,w = img1.shape[:2]
pts = np.float32([ [0,0],[0,h-1],[w-1,h-1],[w-1,0] ]).reshape(-1,1,2)
dst = cv2.perspectiveTransform(pts,M)
dst = np.int32(dst)
offset = np.array([w,0])
dst += offset
result_img = cv2.polylines(img2,[dst],True,(255,0,0),3, cv2.LINE_AA)
result_img[offset[1]:h+offset[1],:w,:] = img1
cv2.imshow("Result", result_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
cv2.error: OpenCV(4.5.5) D:\a\opencv-python\opencv-python\opencv\modules\core\src\matmul.dispatch.cpp:550: error: (-215:Assertion failed) scn + 1 == m.cols in function 'cv::perspectiveTransform']
这个错误可能是由于`pts`的维度不正确导致的。请将其转换为形状为(N, 1, 2)的三维numpy数组,如下所示:
```python
import numpy as np
# 计算图像2在图像1中的偏移量
h, w, _ = img2.shape
pts = [[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]
pts = np.array(pts).reshape(-1, 1, 2)
pts = cv2.perspectiveTransform(pts, H)
x_offset = int(min(pts[:, 0, 0]))
y_offset = int(min(pts[:, 0, 1]))
```
这里使用`reshape`将`pts`转换为形状为(N, 1, 2)的三维numpy数组,以便与cv2.perspectiveTransform函数的参数类型匹配。
阅读全文
相关推荐
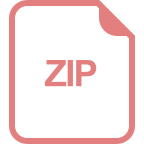
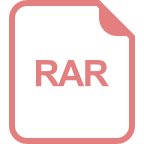
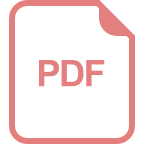
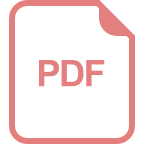
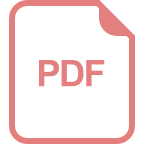
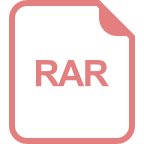
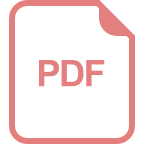







