C++利用顺序栈将10进制数转换为16进制数
时间: 2023-11-25 19:08:21 浏览: 88
以下是使用顺序栈将10进制数转换为16进制数的C++代码:
```c++
#include <iostream>
using namespace std;
// 定义顺序栈结构体
#define MaxSize 100
typedef struct {
int data[MaxSize];
int top;
} SqStack;
// 初始化栈
void InitStack(SqStack &S) {
S.top = -1;
}
// 判断栈是否为空
bool StackEmpty(SqStack S) {
if (S.top == -1) {
return true;
}
return false;
}
// 进栈
bool Push(SqStack &S, int x) {
if (S.top == MaxSize-1) {
return false; // 栈满,无法进栈
}
S.top++;
S.data[S.top] = x;
return true;
}
// 出栈
bool Pop(SqStack &S, int &x) {
if (S.top == -1) {
return false; // 栈空,无法出栈
}
x = S.data[S.top];
S.top--;
return true;
}
// 将10进制数转换为16进制数
void DecimalToHex(int n) {
SqStack S;
InitStack(S);
while (n) {
Push(S, n%16); // 将余数进栈
n /= 16;
}
while (!StackEmpty(S)) {
int x;
Pop(S, x); // 出栈
if (x < 10) {
cout << x;
} else {
cout << (char)(x-10+'A'); // 将10~15转换为A~F输出
}
}
}
int main() {
int n;
cout << "请输入一个10进制整数:";
cin >> n;
cout << "转换为16进制为:";
DecimalToHex(n);
cout << endl;
return 0;
}
```
使用顺序栈的思路是:先将10进制数每次除以16,将余数压入栈中,最后将栈中元素依次出栈并转换为16进制数输出。
阅读全文
相关推荐
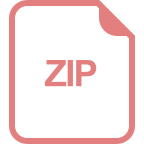


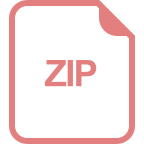
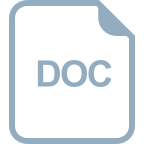









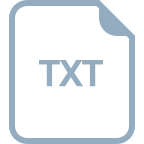
