std::search
时间: 2023-09-06 18:14:16 浏览: 103
std::search is a function in the C++ standard library that searches for a subsequence within a given sequence.
Syntax:
```
template<class ForwardIt1, class ForwardIt2>
ForwardIt1 search(ForwardIt1 first1, ForwardIt1 last1, ForwardIt2 first2, ForwardIt2 last2);
```
Parameters:
- first1, last1: Forward iterators to the initial and final positions of the sequence to search in.
- first2, last2: Forward iterators to the initial and final positions of the subsequence to search for.
Return value:
- Iterator to the first element of the first occurrence of the subsequence in the sequence. If the subsequence is not found, returns last1.
Example:
```
#include <iostream>
#include <algorithm>
#include <vector>
int main()
{
std::vector<int> v {1, 2, 3, 4, 5, 6, 7, 8, 9};
std::vector<int> sub {4, 5, 6};
auto result = std::search(v.begin(), v.end(), sub.begin(), sub.end());
if (result != v.end())
{
std::cout << "Subsequence found starting at index " << std::distance(v.begin(), result) << '\n';
}
else
{
std::cout << "Subsequence not found\n";
}
return 0;
}
```
Output:
```
Subsequence found starting at index 3
```
阅读全文
相关推荐
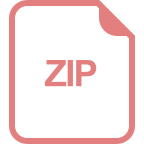
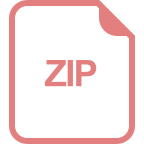
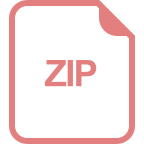















