写一个串口2的发送上位机函数
时间: 2023-07-29 15:11:50 浏览: 47
当然,我可以帮你写一个串口2的发送上位机函数。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int init_serial(const char* port) {
int fd = open(port, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("open_port: Unable to open serial port");
return -1;
}
struct termios options;
tcgetattr(fd, &options);
// 设置波特率为9600
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
// 设置数据位,停止位和校验位
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
// 使能读取
options.c_cflag |= CREAD;
// 设置为原始模式
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
// 禁用软件流控制
options.c_iflag &= ~(IXON | IXOFF | IXANY);
// 禁用硬件流控制
options.c_cflag &= ~CRTSCTS;
// 设置超时
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
// 清空输入输出缓冲区
tcflush(fd, TCIFLUSH);
// 应用设置
tcsetattr(fd, TCSANOW, &options);
return fd;
}
int send_data(int fd, const char* data, int size) {
int count = write(fd, data, size);
if (count < 0) {
perror("write failed");
return -1;
}
return count;
}
int main() {
const char* port = "/dev/ttyS1";
int fd = init_serial(port);
if (fd == -1) {
return 1;
}
const char* data = "Hello, world!";
int size = strlen(data);
int count = send_data(fd, data, size);
if (count == -1) {
return 1;
}
printf("Data sent successfully!\n");
close(fd);
return 0;
}
```
请注意,这只是一个示例代码,你需要根据你的实际情况进行修改。在上面的代码中,`init_serial`函数用于初始化串口,并返回一个文件描述符。`send_data`函数用于发送数据。你可以将要发送的数据存储在`data`数组中,并通过调用`send_data`函数来发送数据。
你可以根据你的需求修改串口的配置参数,比如波特率、数据位、停止位、校验位等。同时,你需要根据你的系统确定正确的串口设备文件路径(比如`/dev/ttyS1`)。
希望这个示例对你有帮助!如果你有任何问题,请随时提问。
相关推荐
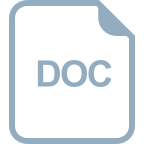
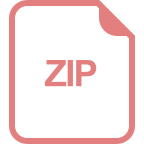
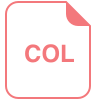













