优化这段代码 class Solution: @staticmethod def Str(iterator): re_strTemp = str() for i in iterator: re_strTemp += i return re_strTemp @staticmethod def extract(indexIn, indexOut, tupStr): return ( tupStr[index] for index in range(indexOut, indexIn + 1) ) @staticmethod def longestLen(index, tupStr_Len): return index if (index < tupStr_Len/2) else ( tupStr_Len - index ) @staticmethod def symmetry(indexA, indexB): ''' About point A symmetry :param indexA: :param indexB: :return: indexB' ''' return indexA * 2 - indexB def longestPalindrome(self, Str: str) -> str: tupStr = tuple(Str) tupStr_Len = len(tupStr) re_strTemp = str() re_strTemp_len = 0 for indexA in range(0, tupStr_Len): indexA_longest = Solution.longestLen( indexA, tupStr_Len) if indexA_longest * 2 > re_strTemp_len: for indexB in range(0, indexA_longest + 1, -1): print(1) indexB_change = Solution.symmetry(indexA, indexB) if (tupStr[indexB] != tupStr[indexB_change]) and( indexB - indexB_change - 1> re_strTemp_len ): print(1) re_strTemp = Solution.Str( Solution.extract(indexB_change + 1, indexB - 1, tupStr) ) re_strTemp_len = ( indexB - indexB_change + 1) break return re_strTemp
时间: 2023-06-08 14:07:32 浏览: 121
可以将对称的部分抽离出来,放在一个函数中,减少代码的冗余。具体做法是:
1. 定义一个新函数来计算字符串的对称部分。
2. 在函数 longestPalindrome 中,使用新函数得到中心点左右对称的字符串,若其长度大于当前最长回文字符串,更新结果。
3. 优化 extract 函数,使用切片来提取子串。
重构后代码如下:
class Solution:
@staticmethod
def symmetry(tupStr, left, right):
'''Symmetry around center at "left" and "right".'''
while left >= 0 and right < len(tupStr) and tupStr[left] == tupStr[right]:
left -= 1
right += 1
return tupStr[left+1:right]
def longestPalindrome(self, Str: str) -> str:
tupStr = tuple(Str)
re_strTemp = ''
for i in range(len(tupStr)):
s1 = self.symmetry(tupStr, i, i) # odd length
s2 = self.symmetry(tupStr, i, i+1) # even length
if len(s1) > len(re_strTemp):
re_strTemp = s1
if len(s2) > len(re_strTemp):
re_strTemp = s2
return re_strTemp
如果想进一步提高效率,可以使用 Manacher 算法,时间复杂度可以优化到 O(N)。
阅读全文
相关推荐
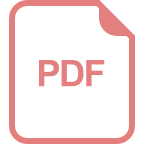
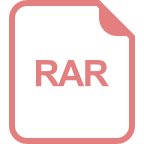
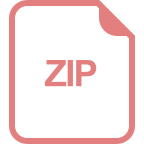















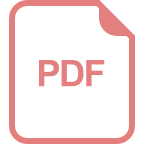
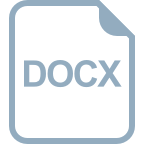