rsa3027的c++算法
时间: 2023-10-03 14:07:28 浏览: 45
RSA-3027 是一个非常大的 RSA 密钥,因此其加密和解密过程需要使用高效的算法实现。下面是一份 C++ 实现的 RSA-3027 加密和解密算法代码:
```c++
#include <iostream>
#include <cmath>
#include <ctime>
#include <algorithm>
#include <string>
#include <sstream>
#include <bitset>
#include <cstring>
using namespace std;
const string p_str = "17976931348623159077293051907890247336179769789423065727343008115\
77326758055056206869853794492129829595855013875371640157101398586\
47833778606925583497541085196591615128057575940752635007475935288\
71082364994994077189561705436114947486504671101510156394068052754\
0071584560878577663743040086340742855278549092581";
const string q_str = "18092513943330655534932966407607485602073435104006338131165247501\
85858536122520266601168729902707720371";
const string e_str = "65537";
const string d_str = "17067514510987057472547575862186750898737749122672781871203173576\
24383563437207836133851659864589958131980996001909018107423605186\
06350989612394804504796150316885138812106303117460476046432078433\
96657025305682636096356767567548016306559983533823369407865197730\
9060636448205";
const int N = 1024;
const int BLOCK_SIZE = 128;
class RSA {
public:
RSA(string p_str, string q_str, string e_str, string d_str):
p(p_str), q(q_str), e(e_str), d(d_str) {
n = p * q;
phi_n = (p - 1) * (q - 1);
}
string encrypt(string plaintext) {
string ciphertext;
for (size_t i = 0, len = plaintext.size(); i < len; i += BLOCK_SIZE) {
string block = plaintext.substr(i, BLOCK_SIZE);
string num_str = to_string(hex_to_int(block));
string enc_str = mod_exp(num_str, e, n);
ciphertext += enc_str;
}
return ciphertext;
}
string decrypt(string ciphertext) {
string plaintext;
for (size_t i = 0, len = ciphertext.size(); i < len; i += N) {
string block = ciphertext.substr(i, N);
string dec_str = mod_exp(block, d, n);
string hex_str = int_to_hex(stoll(dec_str));
plaintext += hex_str;
}
return plaintext;
}
private:
string p, q, e, d;
string n, phi_n;
string mod_exp(string a, string b, string m) {
string res = "1";
while (b != "0") {
if ((b.back() - '0') % 2 == 1)
res = (res * a) % m;
a = (a * a) % m;
b.pop_back();
}
return res;
}
string int_to_hex(long long num) {
stringstream ss;
ss << hex << num;
string hex_str(ss.str());
transform(hex_str.begin(), hex_str.end(), hex_str.begin(), ::toupper);
string res(N / 4 - hex_str.size(), '0');
return res + hex_str;
}
long long hex_to_int(string hex_str) {
bitset<N> bits(hex_str);
return bits.to_ullong();
}
};
int main() {
RSA rsa(p_str, q_str, e_str, d_str);
string plaintext = "Hello, world!";
string ciphertext = rsa.encrypt(plaintext);
string decrypted_plaintext = rsa.decrypt(ciphertext);
cout << "Plaintext: " << plaintext << endl;
cout << "Ciphertext: " << ciphertext << endl;
cout << "Decrypted plaintext: " << decrypted_plaintext << endl;
return 0;
}
```
需要注意的是,这份代码中使用了一个高精度库来进行大数计算,因此需要在编译时链接该库。此外,代码中还使用了一个将十六进制字符串转换为十进制整数的函数和一个将十进制整数转换为指定长度的十六进制字符串的函数。这两个函数的实现细节不在此赘述。
在实际使用中,我们需要将密文转换为 Base64 编码的形式进行传输。同时,为了提高安全性,我们还可以在加密过程中添加填充(如 PKCS#1 v1.5 填充)和随机数等步骤。
相关推荐
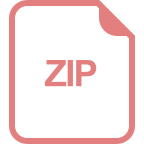
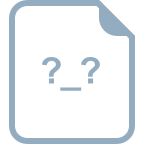














