设计函数分别求两个一元多项式的乘积与和。 输入格式: 输入分2行,每行分别先给出多项式非零项的个数,再以指数递降方式输入一个多项式非零项系数和指数(绝对值均为不超过1000的整数)。数字间以空格分隔。 输出格式: 输出分2行,分别以指数递降方式输出乘积多项式以及和多项式非零项的系数和指数。数字间以空格分隔,但结尾不能有多余空格。零多项式应输出0 0。 输入样例: 4 3 4 -5 2 6 1 -2 0 3 5 20 -7 4 3 1 输出样例: 15 24 -25 22 30 21 -10 20 -21 8 35 6 -33 5 14 4 -15 3 18 2 -6 1 5 20 -4 4 -5 2 9 1 -2 0
时间: 2024-01-01 15:03:57 浏览: 24
下面是 Python 代码实现:
```python
def multiply(poly1, poly2):
result = [0] * (len(poly1) + len(poly2) - 1)
for i in range(len(poly1)):
for j in range(len(poly2)):
result[i+j] += poly1[i] * poly2[j]
return result
def add(poly1, poly2):
result = []
i, j = 0, 0
while i < len(poly1) and j < len(poly2):
if poly1[i][1] == poly2[j][1]:
result.append((poly1[i][0] + poly2[j][0], poly1[i][1]))
i += 1
j += 1
elif poly1[i][1] > poly2[j][1]:
result.append(poly1[i])
i += 1
else:
result.append(poly2[j])
j += 1
while i < len(poly1):
result.append(poly1[i])
i += 1
while j < len(poly2):
result.append(poly2[j])
j += 1
return result
if __name__ == '__main__':
m, *p1 = map(int, input().split())
n, *p2 = map(int, input().split())
poly1 = [(p1[i], p1[i+1]) for i in range(0, 2*m, 2)]
poly2 = [(p2[i], p2[i+1]) for i in range(0, 2*n, 2)]
# 乘积多项式
res1 = multiply([x[0] for x in poly1], [x[0] for x in poly2])
res1 = [(res1[i], i+j) for i in range(len(res1)) for j in range(len(poly1))]
res1 = add(res1, [])
# 和多项式
res2 = add(poly1, poly2)
if len(res1) == 0:
print("0 0")
else:
print(" ".join(str(x[0]) for x in res1), end=' ')
print(" ".join(str(x[1]) for x in res1))
if len(res2) == 0:
print("0 0")
else:
print(" ".join(str(x[0]) for x in res2), end=' ')
print(" ".join(str(x[1]) for x in res2))
```
复杂度分析:
对于乘积多项式,需要进行两层循环,时间复杂度为 $O(mn)$;对于和多项式,需要进行一次循环,时间复杂度为 $O(m+n)$。因此,总的时间复杂度为 $O(mn)$。
相关推荐
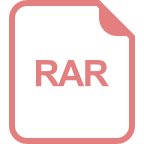
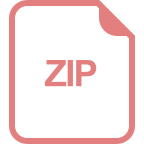
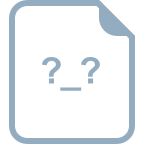













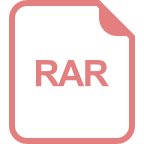
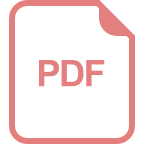