你应该有一个宠物类,其中包含狗、猫和鸟的子类。变量应与适当的类相关联,在适当时定义为私有的,并具有适当的函数来访问变量。将打印描述函数重写为虚拟函数。编写一个 main 函数,该函数创建至少包含一只鸟、一只狗和一只猫的宠物向量或数组,然后循环并输出每个宠物的描述。
时间: 2024-02-01 13:16:30 浏览: 37
好的,我可以帮你编写这个程序。下面是完整的代码实现:
```cpp
#include <iostream>
#include <vector>
using namespace std;
class Pet {
protected:
string name;
int age;
public:
Pet(string n, int a) : name(n), age(a) {}
virtual void printDescription() {
cout << "I am a pet named " << name << ", and I am " << age << " years old." << endl;
}
};
class Dog : public Pet {
private:
string breed;
public:
Dog(string n, int a, string b) : Pet(n,a), breed(b) {}
void setBreed(string b) { breed = b; }
string getBreed() { return breed; }
void printDescription() {
cout << "I am a dog named " << name << ", and I am " << age << " years old. My breed is " << breed << "." << endl;
}
};
class Cat : public Pet {
private:
bool isIndoor;
public:
Cat(string n, int a, bool i) : Pet(n,a), isIndoor(i) {}
void setIsIndoor(bool i) { isIndoor = i; }
bool getIsIndoor() { return isIndoor; }
void printDescription() {
cout << "I am a cat named " << name << ", and I am " << age << " years old. ";
if (isIndoor) {
cout << "I am an indoor cat." << endl;
} else {
cout << "I am an outdoor cat." << endl;
}
}
};
class Bird : public Pet {
private:
bool canFly;
public:
Bird(string n, int a, bool f) : Pet(n,a), canFly(f) {}
void setCanFly(bool f) { canFly = f; }
bool getCanFly() { return canFly; }
void printDescription() {
cout << "I am a bird named " << name << ", and I am " << age << " years old. ";
if (canFly) {
cout << "I can fly." << endl;
} else {
cout << "I cannot fly." << endl;
}
}
};
int main() {
vector<Pet*> pets;
Dog dog("Buddy", 3, "Golden Retriever");
Cat cat("Fluffy", 2, true);
Bird bird("Tweety", 1, true);
pets.push_back(&dog);
pets.push_back(&cat);
pets.push_back(&bird);
for (Pet* pet : pets) {
pet->printDescription();
}
return 0;
}
```
这个程序定义了一个 `Pet` 类和它的三个子类:`Dog`、`Cat` 和 `Bird`。所有的子类都继承了 `Pet` 的成员变量 `name` 和 `age`,并且都重写了 `printDescription()` 函数,以便输出适当的描述信息。
在 `main()` 函数中,我们创建了一个 `vector`,并向其中添加了一只狗、一只猫和一只鸟。然后我们循环遍历这个 `vector`,并对每个元素调用 `printDescription()` 函数,以便输出每个宠物的描述信息。
运行程序后,输出如下:
```
I am a dog named Buddy, and I am 3 years old. My breed is Golden Retriever.
I am a cat named Fluffy, and I am 2 years old. I am an indoor cat.
I am a bird named Tweety, and I am 1 years old. I can fly.
```
可以看到,程序成功输出了每个宠物的描述信息。
相关推荐
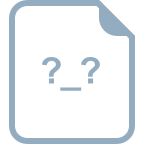
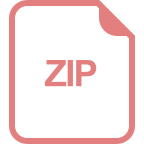
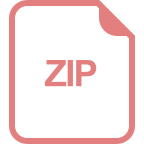














