ffmpeg如何将一个AVFrame的数据进行重采样 c++
时间: 2024-02-17 18:04:14 浏览: 25
在使用FFmpeg进行音视频处理时,重采样是一个常见的操作,可以使用libswresample库来进行重采样。
下面是一个示例代码,用于将一个AVFrame的数据进行重采样:
```c++
#include <iostream>
#include <string>
#include <cstring>
#include <cstdlib>
#include <cstdio>
#include <cmath>
extern "C"
{
#include <libavutil/opt.h>
#include <libavutil/channel_layout.h>
#include <libavutil/samplefmt.h>
#include <libswresample/swresample.h>
}
#define MAX_AUDIO_FRAME_SIZE 192000
int main(int argc, char* argv[])
{
// 输入音频参数
int in_sample_rate = 44100;
int in_channels = 2;
AVSampleFormat in_sample_fmt = AV_SAMPLE_FMT_FLTP;
int in_channel_layout = av_get_default_channel_layout(in_channels);
// 输出音频参数
int out_sample_rate = 48000;
int out_channels = 2;
AVSampleFormat out_sample_fmt = AV_SAMPLE_FMT_S16;
int out_channel_layout = av_get_default_channel_layout(out_channels);
// 初始化重采样器
SwrContext* swr_ctx = swr_alloc_set_opts(nullptr,
out_channel_layout, out_sample_fmt, out_sample_rate,
in_channel_layout, in_sample_fmt, in_sample_rate, 0, nullptr);
if (!swr_ctx)
{
std::cout << "Failed to allocate SwrContext." << std::endl;
return -1;
}
// 初始化重采样器
if (swr_init(swr_ctx) < 0)
{
std::cout << "Failed to initialize SwrContext." << std::endl;
swr_free(&swr_ctx);
return -1;
}
// 分配输入帧和输出帧
AVFrame* in_frame = av_frame_alloc();
if (!in_frame)
{
std::cout << "Failed to allocate AVFrame." << std::endl;
return -1;
}
AVFrame* out_frame = av_frame_alloc();
if (!out_frame)
{
std::cout << "Failed to allocate AVFrame." << std::endl;
av_frame_free(&in_frame);
return -1;
}
// 设置输入帧参数
in_frame->format = in_sample_fmt;
in_frame->channel_layout = in_channel_layout;
in_frame->sample_rate = in_sample_rate;
// 分配输入帧数据
if (av_frame_get_buffer(in_frame, 0) < 0)
{
std::cout << "Failed to allocate input frame data." << std::endl;
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
// 分配输出帧数据
int out_nb_samples = av_rescale_rnd(in_frame->nb_samples,
out_sample_rate, in_sample_rate, AV_ROUND_UP);
out_frame->format = out_sample_fmt;
out_frame->channel_layout = out_channel_layout;
out_frame->sample_rate = out_sample_rate;
out_frame->nb_samples = out_nb_samples;
if (av_frame_get_buffer(out_frame, 0) < 0)
{
std::cout << "Failed to allocate output frame data." << std::endl;
av_frame_free(&in_frame);
av_frame_free(&out_frame);
return -1;
}
// 填充输入帧数据
// 这里的data和linesize要根据输入格式进行设置
float* in_data = reinterpret_cast<float*>(in_frame->data[0]);
int in_linesize = in_channels * in_frame->nb_samples * sizeof(float);
memset(in_data, 0, in_linesize);
for (int i = 0; i < in_frame->nb_samples; ++i)
{
for (int j = 0; j < in_channels; ++j)
{
in_data[j + i * in_channels] = sin(i * 2 * M_PI * 440.0 / in_sample_rate);
}
}
// 进行重采样
uint8_t* out_data = out_frame->data[0];
int out_linesize = out_channels * out_nb_samples * sizeof(int16_t);
int out_samples = swr_convert(swr_ctx, &out_data, out_nb_samples,
const_cast<const uint8_t**>(in_frame->data), in_frame->nb_samples);
// 打印输出帧的数据
for (int i = 0; i < out_channels * out_samples; ++i)
{
std::cout << static_cast<int16_t*>(out_data)[i] << std::endl;
}
// 释放资源
av_frame_free(&in_frame);
av_frame_free(&out_frame);
swr_free(&swr_ctx);
return 0;
}
```
这个示例代码中,首先初始化了重采样器,然后分配了输入帧和输出帧。接着填充了输入帧数据,这里使用了一个简单的正弦波作为输入数据。最后调用swr_convert函数进行重采样,输出的数据存储在out_data中。
需要注意的是,这里的data和linesize要根据输入格式进行设置,否则会导致重采样失败。此外,如果输入帧和输出帧的格式不同,还需要进行数据类型转换,例如从浮点型到整型。
相关推荐
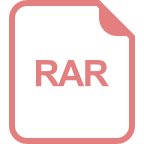














