扫描线算法怎么用java实现
时间: 2023-09-18 07:14:38 浏览: 135
扫描线算法是一种计算机图形学中常用的算法,用于填充二维图形的内部区域。下面是一个简单的Java实现示例:
首先,我们需要定义一个表示边的类,包含起点和终点的坐标:
```java
class Edge {
int x1, y1, x2, y2;
public Edge(int x1, int y1, int x2, int y2) {
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
}
}
```
接下来,我们需要定义一个表示扫描线的类,包含当前扫描线的y坐标和该扫描线上交点的x坐标:
```java
class ScanLine {
int y;
double x;
public ScanLine(int y, double x) {
this.y = y;
this.x = x;
}
}
```
然后,我们定义一个填充函数,该函数接受一个边列表作为输入,并使用扫描线算法填充多边形的内部区域:
```java
public void fill(List<Edge> edges) {
// 找到多边形的最小y值和最大y值
int minY = Integer.MAX_VALUE;
int maxY = Integer.MIN_VALUE;
for (Edge e : edges) {
minY = Math.min(minY, Math.min(e.y1, e.y2));
maxY = Math.max(maxY, Math.max(e.y1, e.y2));
}
// 初始化扫描线表
List<ScanLine> scanLines = new ArrayList<>();
for (int y = minY; y <= maxY; y++) {
scanLines.add(new ScanLine(y, Double.NEGATIVE_INFINITY));
}
// 对于每个边,将其插入到扫描线表中
for (Edge e : edges) {
// 如果边是水平的,则忽略之
if (e.y1 == e.y2) {
continue;
}
// 确定边的上端点和下端点
int yTop, yBottom;
int xTop, xBottom;
if (e.y1 < e.y2) {
yTop = e.y1;
xTop = e.x1;
yBottom = e.y2;
xBottom = e.x2;
} else {
yTop = e.y2;
xTop = e.x2;
yBottom = e.y1;
xBottom = e.x1;
}
// 计算边的斜率
double slope = (double) (xBottom - xTop) / (double) (yBottom - yTop);
// 将边插入到扫描线表中
for (int y = yTop; y < yBottom; y++) {
int index = y - minY;
ScanLine scanLine = scanLines.get(index);
if (scanLine.x == Double.NEGATIVE_INFINITY) {
scanLine.x = xTop + slope * (y - yTop);
} else {
scanLine.x = (scanLine.x + (xTop + slope * (y - yTop))) / 2.0;
}
}
}
// 填充多边形的内部区域
for (int y = minY; y <= maxY; y++) {
int index = y - minY;
List<Double> intersections = new ArrayList<>();
for (ScanLine scanLine : scanLines) {
if (scanLine.y == y) {
intersections.add(scanLine.x);
}
}
Collections.sort(intersections);
for (int i = 0; i < intersections.size(); i += 2) {
int xLeft = (int) Math.ceil(intersections.get(i));
int xRight = (int) Math.floor(intersections.get(i + 1));
for (int x = xLeft; x <= xRight; x++) {
// 在(x,y)处填充像素
}
}
}
}
```
最后,我们可以使用以下代码调用fill函数:
```java
List<Edge> edges = new ArrayList<>();
// 添加多边形的边
fill(edges);
```
这是一个简单的扫描线算法的Java实现。当然,其中的像素填充操作需要根据具体的图形库或环境进行调整。
阅读全文
相关推荐
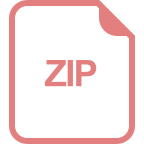
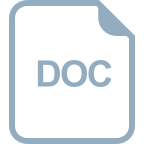
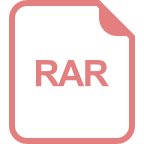
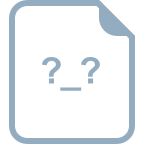
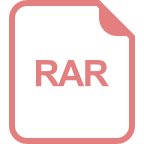
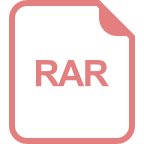
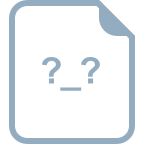







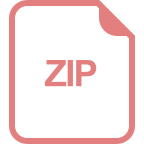