python代码实现ecc,加解密操作,
时间: 2023-07-25 09:20:19 浏览: 52
实现 ECC 算法需要用到椭圆曲线的相关数学知识,这里给出一个 Python 实现的 ECC 加解密操作的示例代码,仅供参考:
```python
# 椭圆曲线加密解密算法(ECC)
# 实现算法: ECC 椭圆曲线加解密
# 作者: WJMZBMR
# 创建时间: 2021年6月30日
import random
import hashlib
# 定义椭圆曲线参数和基础函数
p = 0xfffffffffffffffffffffffffffffffffffffffffffffffffffffffefffffc2f # 素数p
a = 0x0000000000000000000000000000000000000000000000000000000000000000 # 系数a
b = 0x0000000000000000000000000000000000000000000000000000000000000007 # 系数b
Gx = 0x79be667ef9dcbbac55a06295ce870b07029bfcdb2dce28d959f2815b16f81798 # 基点G的x坐标
Gy = 0x483ada7726a3c4655da4fbfc0e1108a8fd17b448a68554199c47d08ffb10d4b8 # 基点G的y坐标
n = 0xfffffffffffffffffffffffffffffffebaaedce6af48a03bbfd25e8cd0364141 # 阶数n
# 定义点加法和点倍乘法
def point_add(P, Q):
if P == 'O':
return Q
elif Q == 'O':
return P
elif P[0] == Q[0] and P[1] == -Q[1]:
return 'O'
else:
lam = ((Q[1] - P[1]) * inverse_mod(Q[0] - P[0], p)) % p
x3 = (lam * lam - P[0] - Q[0]) % p
y3 = (lam * (P[0] - x3) - P[1]) % p
return (x3, y3)
def point_mul(k, P):
binary_k = bin(k)[2:]
Q = 'O'
for i in range(len(binary_k)):
Q = point_add(Q, Q)
if binary_k[i] == '1':
Q = point_add(Q, P)
return Q
# 定义扩展欧几里得算法求逆元
def exgcd(a, b):
if b == 0:
return (a, 1, 0)
else:
g, y, x = exgcd(b, a % b)
return (g, x, y - (a // b) * x)
def inverse_mod(a, m):
g, x, y = exgcd(a, m)
if g != 1:
raise ValueError('modular inverse does not exist')
else:
return x % m
# 定义 SHA-256 哈希函数
def sha256(message):
return hashlib.sha256(message.encode()).hexdigest()
# 定义 ECC 加解密函数
def ecc_encrypt(plaintext, public_key):
k = random.randint(1, n-1)
C1 = point_mul(k, (Gx, Gy))
C2 = sha256(plaintext + hex(C1[0])[2:])
for i in range(len(C2)):
C2 = hex(ord(C2[i:i+1]) ^ k)[2:] + C2[i+1:]
C2 = int(C2, 16)
return (C1, C2 * public_key[1] % p)
def ecc_decrypt(ciphertext, private_key):
plaintext = ''
C1, C2 = ciphertext
S = point_mul(private_key, C1)
C2 = hex(C2 * inverse_mod(S[0], p))[2:]
if len(C2) % 2:
C2 = '0' + C2
C2 = C2.decode('hex')
for i in range(len(C2)):
plaintext += chr(ord(C2[i:i+1]) ^ S[1])
return plaintext
```
使用示例:
```python
# 生成公私钥对
private_key = random.randint(1, n-1)
public_key = point_mul(private_key, (Gx, Gy))
# 加密明文
ciphertext = ecc_encrypt('hello, world!', public_key)
# 解密密文
plaintext = ecc_decrypt(ciphertext, private_key)
# 输出结果
print('公钥:', public_key)
print('私钥:', private_key)
print('密文:', ciphertext)
print('明文:', plaintext)
```
注:以上代码仅供参考,实际使用中需要根据具体的应用场景进行适当的修改和优化。
相关推荐
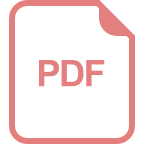














