python代码实现安全的ecc实现
时间: 2023-08-05 08:28:12 浏览: 183
在Python中,可以使用`cryptography`库来实现安全的ECC。
以下是一个简单的示例,用于生成公私钥对、加密和解密数据:
```python
from cryptography.hazmat.primitives.asymmetric import ec
from cryptography.hazmat.primitives import serialization, hashes
from cryptography.hazmat.primitives.ciphers.aead import AESGCM
# 生成椭圆曲线私钥
private_key = ec.generate_private_key(ec.SECP256R1())
# 生成公钥
public_key = private_key.public_key()
# 序列化私钥和公钥
private_key_bytes = private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
)
public_key_bytes = public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
# 加密数据
data = b"Hello, world!"
aesgcm = AESGCM(hashes.SHA256().digest())
nonce = aesgcm.generate_nonce()
encrypted_data = aesgcm.encrypt(nonce, data, None)
# 解密数据
decrypted_data = aesgcm.decrypt(nonce, encrypted_data, None)
print("Private key: ", private_key_bytes)
print("Public key: ", public_key_bytes)
print("Encrypted data: ", encrypted_data)
print("Decrypted data: ", decrypted_data)
```
这个示例使用了SECP256R1椭圆曲线。它生成了一个私钥和一个公钥,并将它们序列化为PEM格式的字节串。然后,它使用AES-GCM加密数据,并使用相同的密钥解密数据。
需要注意的是,在实际应用中,还需要考虑其他安全因素,例如密钥管理、输入验证、输出处理等。
相关推荐
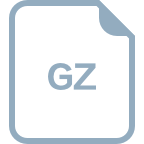
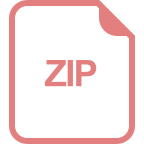














