ecc的python代码实现
时间: 2024-12-26 12:17:47 浏览: 7
### ECC 的 Python 实现
#### 定义椭圆曲线类
为了实现 ECC 加密算法,首先定义一个表示椭圆曲线的类 `EllipticCurve`。
```python
class EllipticCurve:
def __init__(self, a, b, p):
"""初始化椭圆曲线参数"""
self.a = a
self.b = b
self.p = p
def is_on_curve(self, point):
"""判断点是否在曲线上"""
if point is None:
return True
x, y = point
return (y * y - x * x * x - self.a * x - self.b) % self.p == 0
```
该类包含了椭圆曲线方程 \(y^2 \equiv x^3 + ax + b\ (\text{mod}\ p)\),并提供了一个方法来验证某个点是否位于这条曲线上[^1]。
#### 点的操作
接着定义一些基本操作,比如点加法和标量乘法:
```python
def add_points(curve, P, Q):
"""两个不同点相加"""
if P is None or Q is None:
return P if Q is None else Q
xp, yp = P
xq, yq = Q
if xp == xq and yp != yq:
return None # 相反数相加得无穷远点
if P == Q:
lam = (3 * xp * xp + curve.a) * pow(2 * yp, -1, curve.p) % curve.p
else:
lam = (yq - yp) * pow(xq - xp, -1, curve.p) % curve.p
xr = (lam * lam - xp - xq) % curve.p
yr = (lam * (xp - xr) - yp) % curve.p
return int(xr), int(yr)
def scalar_multiply(curve, k, P):
"""标量乘法:k*P"""
result = None
temp = P
while k:
if k & 1:
result = add_points(curve, result, temp)
temp = add_points(curve, temp, temp)
k >>= 1
return result
```
这里实现了两种重要的运算——点加法以及基于双倍-累加策略的快速幂次计算方式来进行标量乘法[^2]。
#### 密钥生成与加密/解密过程
最后展示如何利用上述工具完成公私钥对创建、消息加密及解密的过程:
```python
import random
from hashlib import sha256
def generate_keys(curve, G, nbits):
"""生成一对随机的公私钥"""
private_key = random.randint(1, 2**(nbits)-1)
public_key = scalar_multiply(curve, private_key, G)
return private_key, public_key
def encrypt_message(message, curve, base_point, recipient_public_key):
"""使用接收者的公钥加密一条消息"""
m = message.encode('utf8')
h = int.from_bytes(sha256(m).digest(), 'big')[:len(m)]
r = random.randint(1, curve.p-1)
c1 = scalar_multiply(curve, r, base_point)
c2 = tuple((h[i] ^ byte) for i, byte in enumerate(scalar_multiply(curve, r, recipient_public_key)))
return c1, ''.join(map(chr, c2))
def decrypt_message(ciphertext_pair, curve, private_key):
"""通过发送者提供的密文对及其自身的私钥恢复原始消息"""
c1, c2_str = ciphertext_pair
c2 = bytes(ord(char) for char in c2_str)
s = scalar_multiply(curve, private_key, c1)
inv_s_x = (-s[0]) % curve.p
decrypted_h = bytearray()
for i in range(len(c2)):
decrypted_h.append(c2[i] ^ inv_s_x)
hash_length = len(decrypted_h)//2
original_hash = decrypted_h[:hash_length]
recovered_msg = decrypted_h[hash_length:]
return recovered_msg.decode('utf8'), original_hash.hex() == sha256(recovered_msg).hexdigest().hex()[:(hash_length<<1)]
curve_params = {
"a": 0,
"b": 7,
"p": 2**256 - 2**32 - 977
}
base_point_G = (
0x79BE667EF9DCBBAC55A06295CE870B07029BFCDB2DCE28D959F2815B16F81798,
0x483ADA7726A3C4655DA4FBFC0E1108A8FD17B448A68554199C47D08FFB10D4B8
)
ecc_curve = EllipticCurve(**curve_params)
private_key_user1, public_key_user1 = generate_keys(ecc_curve, base_point_G, 256)
_, public_key_user2 = generate_keys(ecc_curve, base_point_G, 256)
message_to_encrypt = "loveyou"
ciphertext = encrypt_message(message_to_encrypt, ecc_curve, base_point_G, public_key_user2)
decrypted_message, verification_status = decrypt_message(ciphertext, ecc_curve, private_key_user1)
print(f"Original Message: {message_to_encrypt}")
print(f"Cipher Text Pair: {ciphertext}")
if verification_status:
print(f"Decrypted Message: {decrypted_message}")
else:
print("Failed to verify the integrity of the decrypted data.")
```
这段代码展示了完整的 ECC 加密流程,包括但不限于密钥对生成、消息编码转换成哈希值形式、实际执行加密逻辑并将结果返回给调用者;同时也提供了相应的解码函数用于验证接收到的数据完整性[^3]。
阅读全文
相关推荐
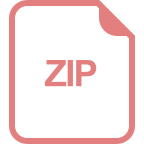
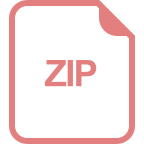
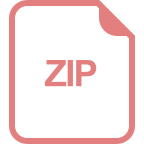

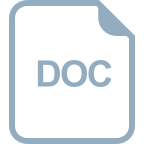
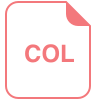
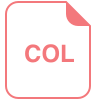
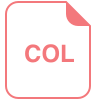










