使用Android studio 做天气预报的代码
时间: 2023-06-16 09:04:31 浏览: 125
以下是使用 Android Studio 实现天气预报的基本代码:
1. 首先,我们需要在 Android Studio 中创建一个新项目。在创建项目时,请确保选择“Empty Activity”作为初始活动。
2. 在 res/layout 文件夹中创建一个新的布局文件,命名为 activity_main.xml。在此布局文件中,我们将添加几个 TextView 和 EditText,以便用户输入城市名称并显示其天气预报。
3. 在 MainActivity.java 中,我们将使用 OpenWeatherMap API 来获取天气预报。请确保您已经在 openweathermap.org 上注册并获取了 API 密钥。
4. 在 MainActivity.java 中,添加以下代码来获取 API 数据:
```
private static String API_KEY = "YOUR_API_KEY";
private static String API_URL = "http://api.openweathermap.org/data/2.5/weather?q=";
public void getWeatherData(String city) {
String url = API_URL + city + "&appid=" + API_KEY;
JsonObjectRequest jsonObjectRequest = new JsonObjectRequest
(Request.Method.GET, url, null, new Response.Listener<JSONObject>() {
@Override
public void onResponse(JSONObject response) {
//处理 API 响应
}
}, new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
//处理错误响应
}
});
//将请求添加到请求队列中
RequestQueue queue = Volley.newRequestQueue(this);
queue.add(jsonObjectRequest);
}
```
5. 在 onResponse 方法中,我们将解析响应并从中提取所需的数据。以下是一个例子:
```
try {
JSONObject mainObject = response.getJSONObject("main");
double temperature = mainObject.getDouble("temp");
double feelsLike = mainObject.getDouble("feels_like");
JSONObject weatherObject = response.getJSONArray("weather").getJSONObject(0);
String description = weatherObject.getString("description");
//更新 UI
TextView temperatureTextView = findViewById(R.id.temperature_textview);
temperatureTextView.setText(temperature + "°C");
TextView feelsLikeTextView = findViewById(R.id.feels_like_textview);
feelsLikeTextView.setText("Feels like " + feelsLike + "°C");
TextView descriptionTextView = findViewById(R.id.description_textview);
descriptionTextView.setText(description);
} catch (JSONException e) {
e.printStackTrace();
}
```
6. 最后,在 MainActivity.java 中,我们将在用户单击“获取天气”按钮时调用 getWeatherData 方法,并传递用户输入的城市名称。
```
Button getWeatherButton = findViewById(R.id.get_weather_button);
getWeatherButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText cityEditText = findViewById(R.id.city_edittext);
String city = cityEditText.getText().toString();
getWeatherData(city);
}
});
```
这是一个简单的天气预报应用程序,您可以在此基础上进行扩展和改进。
相关推荐
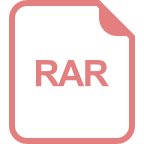














