Python中调用一个接受cv::Mat数据的C++函数
时间: 2024-03-12 14:45:42 浏览: 22
在 Python 中调用一个接受 `cv::Mat` 数据的 C++ 函数,可以使用 `Boost.Python` 库。
假设有如下的 C++ 代码:
```cpp
#include <opencv2/opencv.hpp>
void process_image(cv::Mat image) {
// 对图像进行处理
// ...
}
```
则需要使用 `Boost.Python` 来将 `process_image` 函数导出到 Python 中。示例代码如下:
```cpp
#include <boost/python.hpp>
#include <opencv2/opencv.hpp>
#include <numpy/arrayobject.h>
using namespace boost::python;
void process_image(cv::Mat image) {
// 对图像进行处理
// ...
}
// 将 cv::Mat 转换为 numpy.ndarray
PyObject* mat_to_ndarray(const cv::Mat& mat) {
npy_intp dims[2] = { mat.rows, mat.cols };
int dtype = NPY_UINT8;
int channels = mat.channels();
if (channels == 1) {
dtype = NPY_UINT8;
} else if (channels == 3) {
dtype = NPY_UINT8;
} else if (channels == 4) {
dtype = NPY_UINT8;
}
PyObject* pyobj = PyArray_SimpleNewFromData(2, dims, dtype, mat.data);
PyObject* capsule = PyCapsule_New((void*)mat.data, NULL, NULL);
PyArray_SetBaseObject((PyArrayObject*)pyobj, capsule);
return pyobj;
}
// 将 numpy.ndarray 转换为 cv::Mat
cv::Mat ndarray_to_mat(PyObject* ndarray) {
PyArrayObject* np_array = (PyArrayObject*)ndarray;
int ndims = PyArray_NDIM(np_array);
npy_intp* dims = PyArray_DIMS(np_array);
int dtype = PyArray_TYPE(np_array);
int channels = (dtype == NPY_UINT8) ? 1 : 3;
if (ndims == 3) {
channels = dims[2];
}
cv::Mat mat(dims[0], dims[1], CV_MAKETYPE(dtype, channels), PyArray_DATA(np_array));
return mat.clone();
}
BOOST_PYTHON_MODULE(my_module) {
import_array(); // 初始化 NumPy
// 导出 process_image 函数
def("process_image", process_image);
// 导出 mat_to_ndarray 函数
def("mat_to_ndarray", mat_to_ndarray);
// 导出 ndarray_to_mat 函数
def("ndarray_to_mat", ndarray_to_mat);
}
```
在 Python 中,可以按照以下方式调用 C++ 函数 `process_image`:
```python
import numpy as np
import cv2
import my_module
# 读取图像
image = cv2.imread("image.jpg")
# 将 cv::Mat 转换为 numpy.ndarray
image_array = my_module.mat_to_ndarray(image)
# 调用 C++ 函数处理图像
my_module.process_image(image_array)
```
注意:在使用 NumPy 数组时,需要先初始化 NumPy,可以使用 `import_array()` 函数进行初始化。同时,为了避免内存释放问题,需要在 `mat_to_ndarray` 函数中使用 `PyCapsule_New` 函数创建一个新的 Python 对象,并将其作为 `numpy.ndarray` 的基础对象。
相关推荐
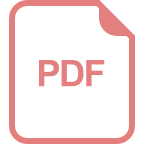
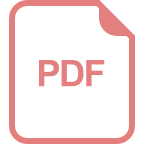














