python读取nifti数据
时间: 2023-10-15 13:02:38 浏览: 210
可以使用Python中的NiBabel (https://nipy.org/nibabel/)库来读取nifti数据。以下是读取nifti文件的示例代码:
```python
import nibabel as nib
# Load the nifti file
nifti_file = nib.load('path/to/nifti/file.nii.gz')
# Get the data array
nifti_data = nifti_file.get_fdata()
# Access the dimensions of the data
nifti_shape = nifti_data.shape
# Access the header information
nifti_header = nifti_file.header
```
在这里,我们导入NiBabel库并加载nifti文件。然后,我们使用“get_fdata()”函数从nifti文件中获取数据数组。使用“shape”函数可以访问数据的尺寸。最后,我们可以访问nifti文件的头信息并将其存储在“nifti_header”变量中。
相关问题
用python实现nifti图像的缩放
在Python中,我们可以使用一些专门处理医学影像数据的库,如` nibabel `和` scikit-image `来读取、操作NIFTI图像文件,并进行缩放。下面是一个简单的例子,展示了如何对NIFTI图像进行尺度变换:
```python
import numpy as np
from nibabel import load
from skimage.transform import resize
# 加载NIFTI图像
img = load('your_image.nii.gz')
data = img.get_fdata() # 获取数据数组
# 定义缩放比例,这里假设是按原尺寸的一半缩小
scale_factor = 0.5
# 对每个维度进行缩放
rescaled_data = resize(data, (int(data.shape[0] * scale_factor), int(data.shape[1] * scale_factor), data.shape[2]))
# 将结果保存到新的NIFTI文件
affine = img.affine
new_img = nib.Nifti1Image(rescaled_data, affine)
new_img.to_filename('scaled_image.nii.gz')
```
在这个示例中,我们首先加载了NIFTI图像,然后使用`skimage.transform.resize`函数对数据进行了归一化缩放。最后,我们将缩放后的数据和原始的 Affine 矩阵组合成一个新的NIFTI图像并保存。
怎样用python实现NIFTI-1文件的三维建模代码
以下是Python实现NIFTI-1文件的三维建模代码示例:
# 导入读取NIFTI-1文件的库
import nibabel as nib
# 加载NIFTI-1文件
nifti_file = nib.load("example.nii.gz")
# 获取NIFTI-1文件数据
nifti_data = nifti_file.get_fdata()
# 获取数据形状
x, y, z, c = nifti_data.shape
# 将NIFTI-1文件数据转化为三维模型数据
model_data = []
for i in range(x):
for j in range(y):
for k in range(z):
voxel = nifti_data[i][j][k]
if voxel > 0:
model_data.append((i, j, k))
# 输出三维模型数据
print(model_data)
注意!此代码仅供参考,具体实现需要根据实际情况进行调整。
阅读全文
相关推荐
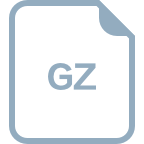















