unity编辑器显示自定义struct
时间: 2023-09-10 14:05:36 浏览: 222
### 回答1:
在Unity编辑器中显示自定义结构体,需要使用Unity的PropertyDrawer功能。您可以通过创建一个继承自PropertyDrawer的类并使用CustomPropertyDrawer特性来实现。
您可以在代码中这样实现:
```
using UnityEngine;
using UnityEditor;
[CustomPropertyDrawer(typeof(YourCustomStruct))]
public class YourCustomStructDrawer : PropertyDrawer
{
public override void OnGUI(Rect position, SerializedProperty property, GUIContent label)
{
// Your custom GUI code here
}
}
```
这样,在Unity编辑器中,您就可以看到您自定义的结构体以符合您的需求的方式显示了。
### 回答2:
在Unity编辑器中显示自定义struct可以通过创建自定义的Editor类来实现。以下是一个基本的示例:
首先,我们需要创建一个名为"CustomStruct"的自定义struct,包含我们希望显示的成员变量和方法。
```csharp
[System.Serializable]
public struct CustomStruct
{
public int intValue;
public float floatValue;
public string stringValue;
public void CustomMethod()
{
// 自定义方法的实现
}
}
```
然后,我们创建一个名为"CustomStructEditor"的自定义Editor类,并继承自UnityEditor类库中的Editor类。
```csharp
using UnityEditor;
using UnityEngine;
[CustomEditor(typeof(MonoBehaviour))]
public class CustomStructEditor : Editor
{
private SerializedProperty intValueProp;
private SerializedProperty floatValueProp;
private SerializedProperty stringValueProp;
private void OnEnable()
{
intValueProp = serializedObject.FindProperty("intValue");
floatValueProp = serializedObject.FindProperty("floatValue");
stringValueProp = serializedObject.FindProperty("stringValue");
}
public override void OnInspectorGUI()
{
serializedObject.Update();
EditorGUILayout.PropertyField(intValueProp);
EditorGUILayout.PropertyField(floatValueProp);
EditorGUILayout.PropertyField(stringValueProp);
serializedObject.ApplyModifiedProperties();
}
}
```
最后,将该自定义Editor类与含有自定义struct的MonoBehaviour脚本关联起来。例如,我们可以将CustomStructEditor类与一个名为"CustomBehaviour"的脚本关联:
```csharp
[CustomEditor(typeof(CustomBehaviour))]
public class CustomBehaviourEditor : Editor
{
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
CustomBehaviour customBehaviour = (CustomBehaviour)target;
EditorGUILayout.Space();
if (GUILayout.Button("Call Custom Method"))
{
customBehaviour.customStruct.CustomMethod();
}
}
}
```
这样,在Unity编辑器中,我们可以像显示其他属性一样显示我们自定义的struct,同时还可以调用自定义方法。
希望这个简单的示例可以帮到您。
### 回答3:
在Unity编辑器中显示自定义的struct(结构体)数据类型,需要通过自定义Unity的自定义编辑器(Custom Editor)来实现。
首先,我们需要在Unity项目中创建一个C#脚本,并在脚本中定义我们需要显示的struct数据类型。例如,我们可以创建一个名为"CustomStruct.cs"的脚本,在其中定义了一个名为"CustomStruct"的struct数据类型。
接下来,我们可以创建一个名为"CustomStructEditor.cs"的自定义编辑器脚本。在该脚本中,我们可以使用Unity的GUI系统来自定义显示struct数据类型的方式。我们可以使用GUILayout或者EditorGUILayout等GUI布局函数来创建自定义的Inspector面板。
在CustomStructEditor.cs中,我们可以重写OnInspectorGUI函数,并在该函数中编写我们希望在Inspector面板中显示的内容。我们可以使用SerializedProperty来访问和修改struct中的成员变量。
例如,我们可以使用GUILayout.Label函数来显示一些文本,使用SerializedProperty.FindPropertyRelative函数来获取struct中的成员变量,并使用 EditorGUILayout.PropertyField 函数来显示每个成员变量。
最后,在CustomStruct.cs脚本的声明前面添加"[CustomEditor(typeof(CustomStructEditor))]"来告诉Unity,我们希望使用我们自定义的编辑器来显示CustomStruct。
总结,通过使用自定义编辑器,我们可以在Unity编辑器中显示自定义的struct数据类型。自定义编辑器使我们能够按照我们的需求来显示和修改struct的成员变量,使开发工作更加高效和灵活。
阅读全文
相关推荐

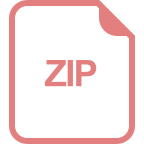
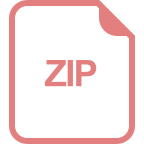
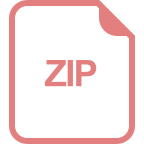
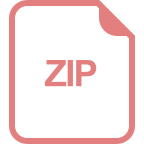
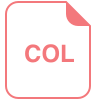
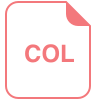
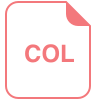
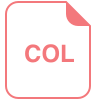
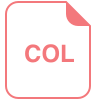
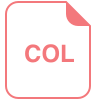
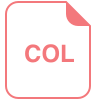
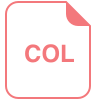
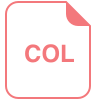
