java编写GUI界面,模拟抢红包游戏,在界面输入红包大小和抢红包人姓名(用逗号隔开),输出系统当前时间以及每个人抢的红包大小,要求每个人抢的红包最少不能少于0.01元,精确到小数点后两位。
时间: 2024-04-01 10:33:52 浏览: 15
好的,这是一个非常有趣的问题!首先,我们需要使用Java的GUI库来构建我们的界面,这里我会使用JavaFX。接着,我们需要使用一些算法来模拟红包的分配过程。以下是一个简单的实现,希望能帮到你:
```java
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
import java.text.DecimalFormat;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class RedPacketGame extends Application {
private final DecimalFormat df = new DecimalFormat("#.00");
private final Random rand = new Random();
private Label timeLabel;
private TextField amountField;
private TextField nameField;
private Button startButton;
private VBox resultBox;
private List<String> names;
private double amount;
@Override
public void start(Stage primaryStage) throws Exception {
timeLabel = new Label();
amountField = new TextField();
nameField = new TextField();
startButton = new Button("开始");
resultBox = new VBox();
names = new ArrayList<>();
VBox root = new VBox();
root.setAlignment(Pos.CENTER);
root.setSpacing(10);
root.getChildren().addAll(timeLabel, amountField, nameField, startButton, resultBox);
Scene scene = new Scene(root, 400, 400);
startButton.setOnAction(event -> startGame());
primaryStage.setTitle("抢红包游戏");
primaryStage.setScene(scene);
primaryStage.show();
}
private void startGame() {
String amountStr = amountField.getText().trim();
String nameStr = nameField.getText().trim();
if (amountStr.isEmpty() || nameStr.isEmpty()) {
showAlert("请输入红包大小和抢红包人姓名");
return;
}
try {
amount = Double.parseDouble(amountStr);
if (amount < 0) {
showAlert("红包大小必须大于零");
return;
}
} catch (NumberFormatException e) {
showAlert("请输入正确的红包大小");
return;
}
String[] nameArray = nameStr.split(",");
for (String name : nameArray) {
name = name.trim();
if (name.isEmpty()) {
showAlert("请输入正确的抢红包人姓名");
return;
}
names.add(name);
}
resultBox.getChildren().clear();
double totalAmount = amount;
for (int i = 0; i < names.size(); i++) {
double min = 0.01;
double max = totalAmount / (names.size() - i);
double money = min + (max - min) * rand.nextDouble();
money = Double.parseDouble(df.format(money));
totalAmount -= money;
String result = String.format("%s 抢到了 %.2f 元", names.get(i), money);
resultBox.getChildren().add(new Label(result));
}
String timeStr = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss").format(LocalDateTime.now());
timeLabel.setText("系统当前时间:" + timeStr);
}
private void showAlert(String message) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle("错误");
alert.setHeaderText(null);
alert.setContentText(message);
alert.showAndWait();
}
public static void main(String[] args) {
launch(args);
}
}
```
在这个代码中,我们创建了一个GUI应用程序,包括一个输入红包大小和抢红包人姓名的文本框,一个开始按钮和一个输出结果的垂直布局。当用户点击开始按钮时,我们会解析输入的红包大小和抢红包人姓名,然后使用一些算法来模拟红包的分配过程,并将结果输出到界面上。
这里,我们使用了一个名为`rand`的随机数生成器来生成随机的红包金额。我们将红包金额限制在0.01元至剩余总金额除以剩余人数之间,以确保每个人至少能够抢到0.01元。最后,我们使用`df`格式化输出结果,以确保金额精确到小数点后两位。
希望这个代码能够帮到你,祝你玩的开心!
相关推荐
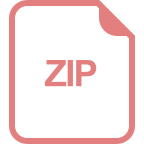
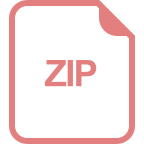
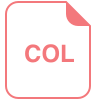
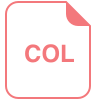
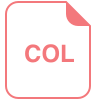
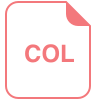
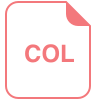









