c语言打开wav处理数据后写入另一个wav例子
时间: 2023-12-04 18:02:45 浏览: 53
好的,以下是一个简单的C语言程序,用于打开WAV文件、处理数据并将其写入另一个WAV文件:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BUFFER_SIZE 4096
typedef struct {
char chunk_id[4];
int chunk_size;
char format[4];
} ChunkHeader;
typedef struct {
char subchunk1_id[4];
int subchunk1_size;
short audio_format;
short num_channels;
int sample_rate;
int byte_rate;
short block_align;
short bits_per_sample;
} WAVHeader;
int main(int argc, char* argv[]) {
char* input_file_path = argv[1];
char* output_file_path = argv[2];
FILE* input_file = fopen(input_file_path, "rb");
if (input_file == NULL) {
perror("Failed to open input file");
exit(EXIT_FAILURE);
}
FILE* output_file = fopen(output_file_path, "wb");
if (output_file == NULL) {
perror("Failed to open output file");
exit(EXIT_FAILURE);
}
// Read WAV header from input file
WAVHeader wav_header;
fread(&wav_header, sizeof(wav_header), 1, input_file);
// Write the same header to output file
fwrite(&wav_header, sizeof(wav_header), 1, output_file);
// Process WAV data
char buffer[BUFFER_SIZE];
while (1) {
size_t bytes_read = fread(buffer, 1, sizeof(buffer), input_file);
if (bytes_read == 0) {
break;
}
// Process buffer here...
// For example, you could invert the audio by subtracting each sample from 32767:
for (int i = 0; i < bytes_read; i += 2) {
short sample = buffer[i] | buffer[i + 1] << 8;
sample = 32767 - sample;
buffer[i] = sample & 0xFF;
buffer[i + 1] = (sample >> 8) & 0xFF;
}
fwrite(buffer, 1, bytes_read, output_file);
}
// Close files
fclose(input_file);
fclose(output_file);
return 0;
}
```
注意,这只是一个简单的示例程序,它没有进行任何错误检查,因此您需要在实际使用中添加错误处理代码。此外,为了让程序更加通用,您可能需要添加更多的参数来指定采样率、位深、通道数等。
相关推荐
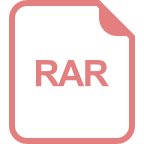














