output = net(x.permute(0, 2, 1)) # 将数据维度转换为(batch_size, in_channels, sequence_length) RuntimeError: permute(sparse_coo): number of dimensions in the tensor input does not match the length of the desired ordering of dimensions i.e. input.dim() = 2 is not equal to len(dims) = 3
时间: 2023-12-12 17:05:29 浏览: 232
这个错误提示是因为 `x` 的维度是 `(batch_size, sequence_length)`,但是在 `net` 的输入中需要将 `in_channels` 这个维度放在第二维。所以需要将 `x` 的维度进行转置,将 `sequence_length` 放在第二维,然后再添加一个维度作为 `in_channels`,即将 `x` 转换为 `(batch_size, in_channels=1, sequence_length)` 的形式,代码如下:
```python
output = net(x.unsqueeze(1)) # 将数据维度转换为(batch_size, in_channels, sequence_length)
```
其中 `unsqueeze(1)` 表示在第二维上增加一个维度。
相关问题
def forward(self, x): fcn_output = self.fcn(x)['out'] fcn_output = fcn_output.permute(0, 2, 3, 1).contiguous().view(-1, fcn_output.size(0), fcn_output.size(1)) transformed_output = self.transformer_encoder(fcn_output) transformed_output = transformed_output.view(fcn_output.size(1), fcn_output.size(2), -1) output = self.linear(transformed_output) return output 改进这段代码
在现有的代码基础上,你可以进行以下改进:
1. 添加激活函数:在输出层之前,可以添加一个激活函数来引入非线性性质。例如,可以在输出层之前使用nn.ReLU()或nn.Sigmoid()等激活函数。
2. 输入维度调整:在转换输出形状为(batch_size, height, width, channels)之前,可以先对fcn_output进行维度调整。例如,可以使用fcn_output.permute(0, 2, 3, 1)将通道维度移至最后。
3. 输出维度调整:根据任务需求,可能需要调整输出的维度。可以使用transformed_output.view(fcn_output.size(0), fcn_output.size(1), fcn_output.size(2), -1)将输出的维度调整为(batch_size, height, width, num_classes)。
4. 损失函数选择:根据任务类型选择合适的损失函数。可以使用交叉熵损失函数(nn.CrossEntropyLoss())来处理分类任务,或者使用适合分割任务的损失函数,如Dice Loss。
5. 训练和评估:添加训练和评估过程的代码,包括数据加载、优化器选择、模型保存等。可以使用PyTorch提供的相关工具来实现。
下面是改进后的代码示例:
```python
def forward(self, x):
fcn_output = self.fcn(x)['out']
fcn_output = fcn_output.permute(0, 2, 3, 1).contiguous()
transformed_output = self.transformer_encoder(fcn_output)
transformed_output = transformed_output.view(fcn_output.size(0), fcn_output.size(2), fcn_output.size(3), -1)
output = self.linear(transformed_output)
output = nn.ReLU()(output) # 添加激活函数
return output
```
同时,记得在训练和评估过程中,根据具体任务需求来选择合适的损失函数和优化器。
希望这些改进能对你有所帮助!如果你还有其他问题,请随时提问。
请使用cuda将nchw的数据转换为nhwc
可以使用以下代码将nchw的数据转换为nhwc:
```python
import torch
# 假设输入数据为nchw格式,大小为(batch_size, channels, height, width)
input_data = torch.randn(batch_size, channels, height, width)
# 将输入数据转换为nhwc格式,大小为(batch_size, height, width, channels)
output_data = input_data.permute(, 2, 3, 1).contiguous()
```
其中,`permute`函数用于交换输入数据的维度顺序,`contiguous`函数用于使输出数据在内存中连续存储。
阅读全文
相关推荐
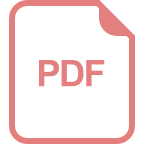
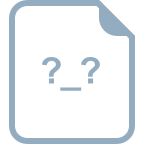
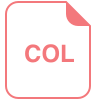
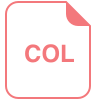
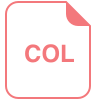






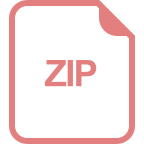
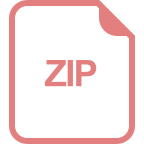