用c语言编写modbus通讯协议
时间: 2023-06-30 15:03:22 浏览: 249
Modbus是一种通用的串行通讯协议,可以用于连接多个设备和传感器,从而实现数据的读取和控制。在C语言中,可以通过串口通讯库来实现Modbus协议的通讯。下面是一个简单的C语言程序,可以实现Modbus RTU协议的读取和写入操作。
```c
#include <stdio.h>
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include <unistd.h>
#include <errno.h>
#include <fcntl.h>
#include <termios.h>
#define DEV_NAME "/dev/ttyS0"
#define BAUD_RATE B9600
#define DATA_BITS 8
#define STOP_BITS 1
#define PARITY_NONE 0
#define SLAVE_ADDR 1
#define FUNC_READ_COILS 0x01
#define FUNC_READ_INPUTS 0x02
#define FUNC_READ_HOLDING_REGS 0x03
#define FUNC_READ_INPUT_REGS 0x04
#define FUNC_WRITE_COIL 0x05
#define FUNC_WRITE_REG 0x06
#define FUNC_WRITE_COILS 0x0F
#define FUNC_WRITE_REGS 0x10
#define READ_COILS 0x0001
#define READ_INPUTS 0x0002
#define READ_HOLDING_REGS 0x0003
#define READ_INPUT_REGS 0x0004
#define WRITE_COIL 0x0005
#define WRITE_REG 0x0006
#define WRITE_COILS 0x000F
#define WRITE_REGS 0x0010
#define OK 0
#define ERR -1
int fd;
int open_serial_port() {
fd = open(DEV_NAME, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
return ERR;
}
struct termios options;
tcgetattr(fd, &options);
options.c_cflag = BAUD_RATE | CS8 | CLOCAL | CREAD;
options.c_iflag = IGNPAR;
options.c_oflag = 0;
options.c_lflag = 0;
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &options);
return OK;
}
int close_serial_port() {
if (close(fd) < 0) {
perror("close");
return ERR;
}
return OK;
}
int read_registers(uint16_t addr, uint16_t count, uint16_t *values) {
uint8_t buf[256];
uint16_t crc;
ssize_t n;
buf[0] = SLAVE_ADDR;
buf[1] = FUNC_READ_INPUT_REGS;
buf[2] = addr >> 8;
buf[3] = addr & 0xFF;
buf[4] = count >> 8;
buf[5] = count & 0xFF;
crc = modbus_crc16(buf, 6);
buf[6] = crc & 0xFF;
buf[7] = crc >> 8;
write(fd, buf, 8);
usleep(10000);
n = read(fd, buf, 256);
if (n < 0) {
perror("read");
return ERR;
}
if (buf[0] != SLAVE_ADDR || buf[1] != FUNC_READ_INPUT_REGS) {
printf("invalid response\n");
return ERR;
}
if (buf[2] != count * 2) {
printf("invalid data length\n");
return ERR;
}
crc = modbus_crc16(buf, 3 + count * 2);
if (crc != 0) {
printf("invalid crc\n");
return ERR;
}
for (int i = 0; i < count; i++) {
values[i] = (buf[3 + i * 2] << 8) | buf[4 + i * 2];
}
return OK;
}
int write_register(uint16_t addr, uint16_t value) {
uint8_t buf[256];
uint16_t crc;
ssize_t n;
buf[0] = SLAVE_ADDR;
buf[1] = FUNC_WRITE_REG;
buf[2] = addr >> 8;
buf[3] = addr & 0xFF;
buf[4] = value >> 8;
buf[5] = value & 0xFF;
crc = modbus_crc16(buf, 6);
buf[6] = crc & 0xFF;
buf[7] = crc >> 8;
write(fd, buf, 8);
usleep(10000);
n = read(fd, buf, 256);
if (n < 0) {
perror("read");
return ERR;
}
if (buf[0] != SLAVE_ADDR || buf[1] != FUNC_WRITE_REG) {
printf("invalid response\n");
return ERR;
}
crc = modbus_crc16(buf, 6);
if (crc != 0) {
printf("invalid crc\n");
return ERR;
}
return OK;
}
uint16_t modbus_crc16(uint8_t *buf, uint16_t len) {
uint16_t crc = 0xFFFF;
for (int i = 0; i < len; i++) {
crc ^= buf[i];
for (int j = 0; j < 8; j++) {
if (crc & 1) {
crc >>= 1;
crc ^= 0xA001;
} else {
crc >>= 1;
}
}
}
return crc;
}
int main() {
uint16_t values[2];
int ret;
if (open_serial_port() != OK) {
printf("failed to open serial port\n");
return 1;
}
ret = read_registers(0, 2, values);
if (ret == OK) {
printf("data1: %d\n", values[0]);
printf("data2: %d\n", values[1]);
} else {
printf("failed to read registers\n");
}
ret = write_register(1, 100);
if (ret == OK) {
printf("write register succeeded\n");
} else {
printf("failed to write register\n");
}
if (close_serial_port() != OK) {
printf("failed to close serial port\n");
return 1;
}
return 0;
}
```
在上面的程序中,首先定义了串口的参数,包括设备名称、波特率、数据位、停止位、校验位等。然后定义了Modbus协议的一些常量,包括读写寄存器的功能码、错误码等。接着定义了打开和关闭串口的函数。
在读取寄存器的函数中,首先构造了Modbus RTU协议的请求报文,然后计算出CRC校验码,并将报文发送到串口中。接着等待一段时间,然后读取串口中的应答报文,解析出其中的数据值,并进行CRC校验。如果校验通过,则将数据值返回给调用函数。
在写入寄存器的函数中,也是构造了Modbus RTU协议的请求报文,并计算出CRC校验码。然后将报文发送到串口中,并等待一段时间,读取应答报文并进行CRC校验。如果校验通过,则写入成功。
最后,在main函数中,先打开串口,然后读取两个寄存器的值,并将其打印出来。接着写入一个寄存器的值,并打印出写入成功的信息。最后关闭串口。
需要注意的是,上面的程序只是一个简单的示例,实际应用中需要根据具体的设备和通讯协议进行修改。同时,还需要进行错误处理和异常情况的处理,例如超时等。
相关推荐













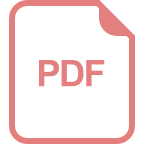
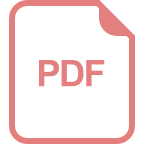