Write a python function proddigits() that inputs a positive integer number and returns the product of digits of that number.
时间: 2024-01-03 14:04:52 浏览: 82
Sure, here's the code for the proddigits() function in Python:
```python
def proddigits(n):
"""Returns the product of digits of a positive integer number n."""
product = 1
while n > 0:
digit = n % 10
product *= digit
n //= 10
return product
```
You can call this function by passing a positive integer number as an argument, like this:
```python
result = proddigits(12345)
print(result) # Output: 120
```
This will calculate the product of digits of the number 12345, which is 1 * 2 * 3 * 4 * 5 = 120.
相关问题
用for loop回答下面问题Write a python function proddigits() that inputs a positive integer number and returns the product of digits of that number. For example, if the input is 422, the function should return 16 (which is equal to 4×2×2 ).
Sure, here is the modified `proddigits()` function that uses a `for` loop to calculate the product of digits:
```python
def proddigits(n):
# Initialize the result
result = 1
# Convert the number to a string for iteration
n_str = str(n)
# Loop through each digit of the number
for digit in n_str:
# Convert the digit back to an integer
digit_int = int(digit)
# Multiply it to the result
result *= digit_int
# Return the final result
return result
```
You can call this function with a positive integer argument to get the product of its digits. For example:
```python
# Test the function
num = 422
print(proddigits(num)) # Output: 16
```
I hope this helps! Let me know if you have any other questions.
我给你一个java题目帮我解答一下:使用JAVA语句进行整数输入输出与简单运算Write programs that read a sequence of integer inputs and print a. The smallest and largest of the inputs. b. The number of even and odd inputs. c. Cumulative totals. For example, if the input is 1 7 2 9, the program should print 1 8 10 19. d. All adjacent duplicates. For example, if the input is 1 3 3 4 5 5 6 6 6 2, the program should print 3 5 6.
答案:import java.util.Scanner;
public class IntegerInputOutput {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int min = Integer.MAX_VALUE;
int max = Integer.MIN_VALUE;
int evenCount = 0;
int oddCount = 0;
int total = 0;
System.out.print("请输入一个整数序列:");
while (sc.hasNextInt()) {
int num = sc.nextInt();
//求最小值
if (num < min)
min = num;
//求最大值
if (num > max)
max = num;
//求偶数个数
if (num % 2 == 0)
evenCount++;
//求奇数个数
if (num % 2 != 0)
oddCount++;
//求累加和
total += num;
}
System.out.println("最小值:" + min);
System.out.println("最大值:" + max);
System.out.println("偶数个数:" + evenCount);
System.out.println("奇数个数:" + oddCount);
System.out.println("累加和:" + total);
//求所有相邻重复的数字
int currNum = 0;
int preNum = 0;
System.out.print("所有相邻重复的数字:");
while (sc.hasNextInt()) {
currNum = sc.nextInt();
if (preNum == currNum)
System.out.print(currNum + " ");
preNum = currNum;
}
}
}
阅读全文
相关推荐












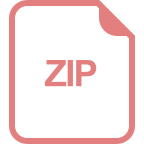