用HTML写一个贪吃蛇小游戏
时间: 2023-02-09 21:09:27 浏览: 351
可以使用 HTML、CSS 和 JavaScript 来编写贪吃蛇小游戏。下面是一个简单的 HTML 结构:
```
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇</title>
</head>
<body>
<canvas id="gameCanvas"></canvas>
</body>
</html>
```
这段代码创建了一个带有 `id` 为 "gameCanvas" 的画布,我们将在其中绘制贪吃蛇游戏。
然后,你可以使用 JavaScript 来编写游戏逻辑。首先,你需要获取 canvas 元素:
```
const canvas = document.getElementById('gameCanvas');
```
然后,你需要设置 canvas 的宽度和高度,并获取其 2D 绘图上下文:
```
canvas.width = 500;
canvas.height = 500;
const ctx = canvas.getContext('2d');
```
接下来,你可以使用 2D 绘图上下文的方法来绘制贪吃蛇和食物,例如 `fillRect` 和 `fillCircle`。
最后,你可以使用 JavaScript 定时器(例如 `setInterval`)来更新游戏状态,并根据玩家的输入(例如键盘事件)来控制贪吃蛇的移动方向。
这是一个非常简单的贪吃蛇小游戏的示例。希望这对你有帮助!
相关问题
用html和javascripe写一个贪吃蛇小游戏
好的,以下是一个简单的贪吃蛇小游戏的 HTML 和 JavaScript 代码:
HTML 代码:
```
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇小游戏</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript 代码(保存为 snake.js 文件):
```
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
var snake = [{x: 10, y: 10}];
var food = {x: 0, y: 0};
var direction = "right";
var score = 0;
function drawSnake() {
ctx.fillStyle = "green";
for (var i = 0; i < snake.length; i++) {
ctx.fillRect(snake[i].x * 10, snake[i].y * 10, 10, 10);
}
}
function drawFood() {
ctx.fillStyle = "red";
ctx.fillRect(food.x * 10, food.y * 10, 10, 10);
}
function generateFood() {
food.x = Math.floor(Math.random() * 40);
food.y = Math.floor(Math.random() * 40);
}
function moveSnake() {
var head = {x: snake[0].x, y: snake[0].y};
if (direction == "right") {
head.x++;
} else if (direction == "left") {
head.x--;
} else if (direction == "up") {
head.y--;
} else if (direction == "down") {
head.y++;
}
snake.unshift(head);
if (head.x == food.x && head.y == food.y) {
score++;
generateFood();
} else {
snake.pop();
}
}
function checkCollision() {
var head = snake[0];
if (head.x < 0 || head.x >= 40 || head.y < 0 || head.y >= 40) {
return true;
}
for (var i = 1; i < snake.length; i++) {
if (head.x == snake[i].x && head.y == snake[i].y) {
return true;
}
}
return false;
}
function update() {
moveSnake();
if (checkCollision()) {
clearInterval(interval);
alert("游戏结束!得分:" + score);
}
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawSnake();
drawFood();
}
generateFood();
var interval = setInterval(update, 100);
document.addEventListener("keydown", function(event) {
if (event.keyCode == 37 && direction != "right") {
direction = "left";
} else if (event.keyCode == 38 && direction != "down") {
direction = "up";
} else if (event.keyCode == 39 && direction != "left") {
direction = "right";
} else if (event.keyCode == 40 && direction != "up") {
direction = "down";
}
});
```
这个小游戏使用 canvas 元素绘制,通过 JavaScript 控制蛇的移动和食物的生成,使用键盘事件监听器控制蛇的方向。
帮我用js写一个贪吃蛇小游戏
好的,以下是一个简单的贪吃蛇小游戏的JS代码,你可以在HTML页面中引用它:
```javascript
// 定义画布大小
var canvasWidth = 600;
var canvasHeight = 600;
// 定义每个正方形格子的大小
var blockSize = 10;
// 计算画布中可以放置多少个正方形格子
var widthInBlocks = canvasWidth/blockSize;
var heightInBlocks = canvasHeight/blockSize;
// 定义画布和上下文
var canvas = document.createElement('canvas');
canvas.width = canvasWidth;
canvas.height = canvasHeight;
document.body.appendChild(canvas);
var ctx = canvas.getContext('2d');
// 定义贪吃蛇初始位置
var snake = {
segments: [[6,4], [5,4], [4,4]],
direction: 'right'
};
// 定义食物初始位置
var food = {
location: [Math.floor(Math.random()*widthInBlocks), Math.floor(Math.random()*heightInBlocks)]
};
// 定义游戏进行与结束的变量
var intervalId;
var gameOver = false;
// 绘制正方形格子
var drawBlock = function(ctx, position) {
var x = position[0] * blockSize;
var y = position[1] * blockSize;
ctx.fillRect(x, y, blockSize, blockSize);
};
// 绘制贪吃蛇
var drawSnake = function(ctx) {
for (var i = 0; i < snake.segments.length; i++) {
drawBlock(ctx, snake.segments[i]);
}
};
// 绘制食物
var drawFood = function(ctx) {
drawBlock(ctx, food.location);
};
// 移动贪吃蛇
var moveSnake = function() {
var head = snake.segments[0];
switch (snake.direction) {
case 'up':
snake.segments.unshift([head[0], head[1] - 1]);
break;
case 'down':
snake.segments.unshift([head[0], head[1] + 1]);
break;
case 'left':
snake.segments.unshift([head[0] - 1, head[1]]);
break;
case 'right':
snake.segments.unshift([head[0] + 1, head[1]]);
break;
}
if (snake.segments[0][0] === food.location[0] && snake.segments[0][1] === food.location[1]) {
food = {
location: [Math.floor(Math.random() * widthInBlocks), Math.floor(Math.random() * heightInBlocks)]
};
} else {
snake.segments.pop();
}
};
// 碰撞检测
var checkCollision = function() {
var head = snake.segments[0];
if (head[0] < 0 || head[0] >= widthInBlocks || head[1] < 0 || head[1] >= heightInBlocks) {
return true;
}
for (var i = 1; i < snake.segments.length; i++) {
if (head[0] === snake.segments[i][0] && head[1] === snake.segments[i][1]) {
return true;
}
}
return false;
};
// 游戏结束
var endGame = function() {
clearInterval(intervalId);
gameOver = true;
ctx.font = 'bold 70px sans-serif';
ctx.fillStyle = '#000';
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
ctx.fillText('Game Over', canvasWidth/2, canvasHeight/2);
};
// 游戏主循环
var gameLoop = function() {
ctx.clearRect(0, 0, canvasWidth, canvasHeight);
drawSnake(ctx);
drawFood(ctx);
moveSnake();
if (checkCollision()) {
endGame();
} else {
intervalId = setTimeout(gameLoop, 100);
}
};
// 开始游戏
intervalId = setTimeout(gameLoop, 100);
// 监听键盘事件
document.addEventListener('keydown', function(event) {
if (event.keyCode === 37 && snake.direction !== 'right') {
snake.direction = 'left';
} else if (event.keyCode === 38 && snake.direction !== 'down') {
snake.direction = 'up';
} else if (event.keyCode === 39 && snake.direction !== 'left') {
snake.direction = 'right';
} else if (event.keyCode === 40 && snake.direction !== 'up') {
snake.direction = 'down';
}
});
```
以上代码可能有些简单,但足以让你了解贪吃蛇小游戏的基本实现原理,你可以在此基础上进行更复杂的开发。
阅读全文
相关推荐
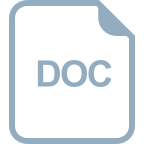
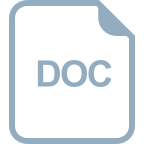
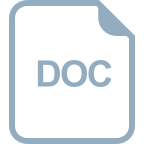












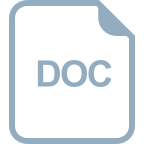
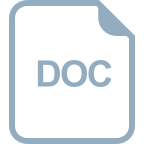