灰度共生矩阵python代码
时间: 2023-07-09 10:41:50 浏览: 48
下面是使用Python实现灰度共生矩阵的示例代码,代码中使用了Python的numpy和opencv库:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('image.png', 0)
# 灰度共生矩阵参数
d = 1 # 距离
theta = [0, np.pi/4, np.pi/2, 3*np.pi/4] # 方向
levels = 256 # 灰度级数目
# 计算灰度共生矩阵
glcm = np.zeros((levels, levels, len(theta)))
for i, angle in enumerate(theta):
offset = np.array([d*np.cos(angle), -d*np.sin(angle)])
for y in range(img.shape[0]):
for x in range(img.shape[1]):
yp = int(round(y + offset[1]))
xp = int(round(x + offset[0]))
if yp >= 0 and yp < img.shape[0] and xp >= 0 and xp < img.shape[1]:
glcm[img[y, x], img[yp, xp], i] += 1
# 归一化灰度共生矩阵
glcm /= np.sum(glcm)
# 计算灰度共生矩阵特征
features = np.zeros((len(theta), 4))
for i in range(len(theta)):
# 能量
features[i, 0] = np.sum(glcm[:,:,i]**2)
# 对比度
features[i, 1] = np.sum((np.arange(levels)[:,np.newaxis,np.newaxis] - np.arange(levels)[np.newaxis,:,np.newaxis])**2 * glcm[:,:,i])
# 相关性
mu_x = np.sum(glcm[:,:,i] * np.arange(levels)[:,np.newaxis])
mu_y = np.sum(glcm[:,:,i] * np.arange(levels)[np.newaxis,:])
sigma_x = np.sqrt(np.sum(glcm[:,:,i] * (np.arange(levels)[:,np.newaxis] - mu_x)**2))
sigma_y = np.sqrt(np.sum(glcm[:,:,i] * (np.arange(levels)[np.newaxis,:] - mu_y)**2))
cov = np.sum(glcm[:,:,i] * (np.arange(levels)[:,np.newaxis] - mu_x) * (np.arange(levels)[np.newaxis,:] - mu_y))
features[i, 2] = cov / (sigma_x * sigma_y)
# 熵
features[i, 3] = -np.sum(glcm[:,:,i] * np.log2(glcm[:,:,i] + 1e-10))
print(features)
```
该代码读取一张灰度图像,并计算其在四个方向上的灰度共生矩阵,然后归一化灰度共生矩阵,并计算每个方向上的能量、对比度、相关性和熵四个特征。最终输出一个4x4的特征矩阵。
相关推荐
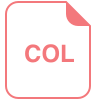













