unity 使用NewTonSoft.json实现字典序列化与反序列化代码
时间: 2024-04-29 14:27:14 浏览: 217
可以使用如下代码实现:
```
using Newtonsoft.Json;
using System.Collections.Generic;
public class MyDictionaryConverter : JsonConverter
{
public override bool CanConvert(Type objectType)
{
return objectType.IsGenericType && objectType.GetGenericTypeDefinition() == typeof(Dictionary<,>);
}
public override void WriteJson(JsonWriter writer, object value, JsonSerializer serializer)
{
var dictionary = (IDictionary)value;
var keyType = dictionary.GetType().GetGenericArguments()[0];
var valueType = dictionary.GetType().GetGenericArguments()[1];
writer.WriteStartObject();
foreach (var key in dictionary.Keys)
{
writer.WritePropertyName(JsonConvert.SerializeObject(key));
serializer.Serialize(writer, dictionary[key]);
}
writer.WriteEndObject();
}
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
{
var dictionary = (IDictionary)Activator.CreateInstance(objectType);
var keyType = objectType.GetGenericArguments()[0];
var valueType = objectType.GetGenericArguments()[1];
while (reader.Read() && reader.TokenType == JsonToken.PropertyName)
{
var propertyName = JsonConvert.DeserializeObject(reader.Value.ToString(), keyType);
var value = serializer.Deserialize(reader, valueType);
dictionary.Add(propertyName, value);
}
return dictionary;
}
}
```
使用方法如下:
```
// 序列化
Dictionary<string, object> dic = new Dictionary<string, object>();
string json = JsonConvert.SerializeObject(dic, new MyDictionaryConverter());
// 反序列化
Dictionary<string, object> result = JsonConvert.DeserializeObject<Dictionary<string, object>>(json, new MyDictionaryConverter());
```
需要注意的是,需要在属性上添加 `[JsonConverter(typeof(MyDictionaryConverter))]` 特性来告诉 `Newtonsoft.Json` 使用上述自定义的 `MyDictionaryConverter` 来进行序列化和反序列化。
阅读全文
相关推荐
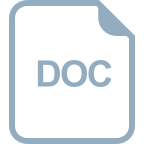
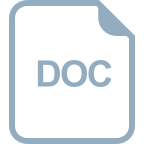
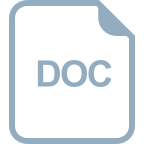

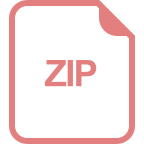
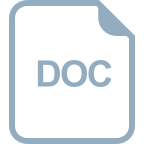
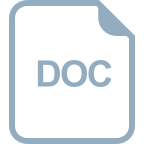







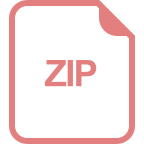
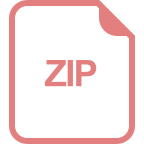
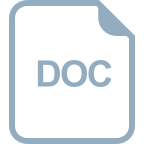
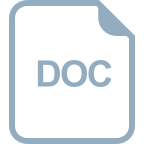

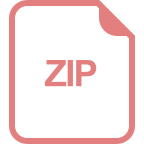