#include<stdio.h> #include<math.h> double fun(double x){ double f,t; int n; /************found*********/ f=1.0+___1___; t=x; n=1; do { n++; /************found**********/ t*=x/___2___; /************found**********/ f+=___3___; } while(fabs(t)>=1e-6); return f; } void main(){ double x,y; scanf("%lf",&x); y=fun(x); printf("%-12.6f",y); }
时间: 2023-07-06 18:37:21 浏览: 98
这段代码是一个求幂级数的C语言程序,通过输入一个实数x,计算并输出1+x+x^2/2!+x^3/3!+...+x^n/n!的值。程序的完整代码如下:
```
#include <stdio.h>
#include <math.h>
double fun(double x){
double f,t;
int n;
f=1.0+x; // 第一项为1+x
t=x;
n=1;
do {
n++;
t*=x/n; // t的更新公式为t*=x/n
f+=t; // f的更新公式为f+=t
} while(fabs(t)>=1e-6); // 当t的绝对值小于1e-6时停止循环
return f;
}
int main(){
double x,y;
scanf("%lf",&x); // 输入要计算的实数x
y=fun(x); // 计算幂级数的值
printf("%-12.6f",y); // 输出结果,保留6位小数
return 0;
}
```
需要填空的部分如下:
1. 第一项的系数为1,第二项的系数为x,因此第一空要填1.0,第二空要填x。
2. t的更新公式为t*=x/n,因此第二空要填n。
3. f的更新公式为f+=t,因此第三空要填t。
希望这个解释对你有所帮助。
相关问题
#include<conio.h> #include<stdio.h> #include<math.h> double fun(int n) { double s, t; int i; s = 1.0;// t = 1.0; for (i = 1; i <= n; i++) { t = t*i/(2 * i + 1); s += t; } return s; } int main() { printf("\n%12.10lf", fun(25)); }
这是一个使用 C 语言编写的程序,其中定义了一个函数 fun,该函数的作用是计算一个数学公式的近似值并返回结果。程序输出了 fun(25) 的结果。
具体来说,该函数的公式为 ∑(i=1 to n) (i!)/(2i+1),其中 n 为函数的参数。在函数中,使用 for 循环计算累加和,每次迭代都计算并累加一项,最终得到近似值并返回。该程序的输出结果为近似值,输出格式为小数,保留了 10 位有效数字。
需要注意的是,该程序使用了头文件 conio.h 和 math.h,前者用于控制台输入输出,后者用于数学计算。这两个头文件在不同的编译环境中可能有所不同,因此需要根据编译环境进行适当的调整。
#include <math.h> #include <stdio.h> double fun(int n) { } main() { int n; double sum; printf("\n\nInput n: "); scanf("%d",&n); sum=fun(n); printf("\n\nsum=%f\n\n",sum); }用C语言
以下是一个简单的程序,可以计算出1到n的平方根倒数之和:
```
#include <stdio.h>
#include <math.h>
double fun(int n) {
double sum = 0.0;
int i;
for (i = 1; i <= n; i++) {
sum += 1.0 / sqrt(i);
}
return sum;
}
int main() {
int n;
double sum;
printf("\n\nInput n: ");
scanf("%d", &n);
sum = fun(n);
printf("\n\nsum=%f\n\n", sum);
return 0;
}
```
函数`fun`接受一个整数`n`作为参数,返回1到n的平方根倒数之和。主函数中输入n,调用fun函数,然后输出结果。
阅读全文
相关推荐















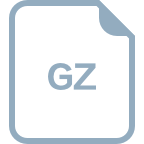