编写非递归遍历算法,实现:给定一颗二叉树的先序遍历序列和中序遍历序列,创建这颗二叉树。 (1)输出二叉树的后序遍历的结点序列。 (2)输出二叉树的叶子结点。 (3)统计二叉树的结点个数。 (4)求二叉树的深度。 (5)输出二叉树指定结点的路径。
时间: 2024-01-23 20:40:25 浏览: 22
非递归遍历算法:
先序遍历:利用栈来实现,先将根节点入栈,然后弹出栈顶元素,输出值并将其右子节点和左子节点分别入栈。重复以上操作直到栈为空。
中序遍历:先将当前节点及其所有左子节点入栈,然后弹出栈顶元素,输出值并将其右子节点入栈,重复以上操作直到栈为空。
后序遍历:需要两个栈来实现,第一个栈按照先序遍历的方式存储节点,第二个栈则逆序存储第一个栈出栈的节点。最后输出第二个栈的元素即为后序遍历结果。
创建二叉树:
根据先序遍历的结果确定根节点,在中序遍历中找到根节点的位置,左边的为左子树,右边的为右子树。递归执行该过程即可。
代码实现:
```
#include <iostream>
#include <stack>
#include <vector>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
TreeNode* buildTree(vector<int>& preorder, vector<int>& inorder) {
if (preorder.empty() || inorder.empty()) return NULL;
int root_val = preorder[0];
TreeNode* root = new TreeNode(root_val);
int i = 0;
while (inorder[i] != root_val) i++;
vector<int> left_pre(preorder.begin() + 1, preorder.begin() + i + 1);
vector<int> left_in(inorder.begin(), inorder.begin() + i);
vector<int> right_pre(preorder.begin() + i + 1, preorder.end());
vector<int> right_in(inorder.begin() + i + 1, inorder.end());
root->left = buildTree(left_pre, left_in);
root->right = buildTree(right_pre, right_in);
return root;
}
vector<int> postorderTraversal(TreeNode* root) {
vector<int> res;
if (!root) return res;
stack<TreeNode*> s1, s2;
s1.push(root);
while (!s1.empty()) {
TreeNode* cur = s1.top();
s1.pop();
s2.push(cur);
if (cur->left) s1.push(cur->left);
if (cur->right) s1.push(cur->right);
}
while (!s2.empty()) {
res.push_back(s2.top()->val);
s2.pop();
}
return res;
}
vector<int> leafNodes(TreeNode* root) {
vector<int> res;
if (!root) return res;
stack<TreeNode*> s;
s.push(root);
while (!s.empty()) {
TreeNode* cur = s.top();
s.pop();
if (!cur->left && !cur->right) res.push_back(cur->val);
if (cur->right) s.push(cur->right);
if (cur->left) s.push(cur->left);
}
return res;
}
int countNodes(TreeNode* root) {
if (!root) return 0;
return countNodes(root->left) + countNodes(root->right) + 1;
}
int maxDepth(TreeNode* root) {
if (!root) return 0;
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
}
bool getPath(TreeNode* root, TreeNode* target, vector<TreeNode*>& path) {
if (!root) return false;
path.push_back(root);
if (root == target) return true;
if (getPath(root->left, target, path) || getPath(root->right, target, path)) return true;
path.pop_back();
return false;
}
vector<TreeNode*> findPath(TreeNode* root, TreeNode* target) {
vector<TreeNode*> path;
getPath(root, target, path);
return path;
}
int main() {
vector<int> preorder = {1, 2, 4, 5, 3, 6, 7};
vector<int> inorder = {4, 2, 5, 1, 6, 3, 7};
TreeNode* root = buildTree(preorder, inorder);
vector<int> postorder = postorderTraversal(root);
vector<int> leaf_nodes = leafNodes(root);
int node_count = countNodes(root);
int depth = maxDepth(root);
TreeNode* target = root->left->right;
vector<TreeNode*> path = findPath(root, target);
cout << "postorder: ";
for (int i = 0; i < postorder.size(); i++) {
cout << postorder[i] << " ";
}
cout << endl;
cout << "leaf nodes: ";
for (int i = 0; i < leaf_nodes.size(); i++) {
cout << leaf_nodes[i] << " ";
}
cout << endl;
cout << "node count: " << node_count << endl;
cout << "depth: " << depth << endl;
cout << "path: ";
for (int i = 0; i < path.size(); i++) {
cout << path[i]->val << " ";
}
cout << endl;
return 0;
}
```
相关推荐
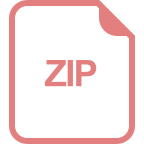
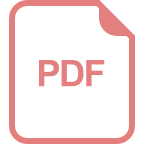
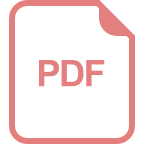














