2.编写非递归遍历算法,实现:给定一棵二叉树的先序遍历序列和中序遍历序列,创建这 棵二叉树。 (1)输出二叉树的后序遍历的结点序列。 (2)输出二叉树的叶子结点。 (3)统计二叉树的结点个数。 (4)求二叉树的深度。 (5)输出二叉树指定结点的路径。
时间: 2024-01-23 12:45:21 浏览: 64
以下是非递归遍历的实现:
```python
class Node:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def build_tree(preorder, inorder):
if not preorder or not inorder:
return None
root = Node(preorder[0])
stack = [root]
inorder_index = 0
for i in range(1, len(preorder)):
current = Node(preorder[i])
parent = stack[-1]
if parent.val != inorder[inorder_index]:
parent.left = current
else:
while stack and stack[-1].val == inorder[inorder_index]:
parent = stack.pop()
inorder_index += 1
parent.right = current
stack.append(current)
return root
def postorder_traversal(root):
if not root:
return []
stack1 = [root]
stack2 = []
while stack1:
node = stack1.pop()
stack2.append(node)
if node.left:
stack1.append(node.left)
if node.right:
stack1.append(node.right)
res = []
while stack2:
res.append(stack2.pop().val)
return res
def get_leaf_nodes(root):
if not root:
return []
stack = [root]
res = []
while stack:
node = stack.pop()
if not node.left and not node.right:
res.append(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return res
def count_nodes(root):
if not root:
return 0
stack = [root]
count = 0
while stack:
node = stack.pop()
count += 1
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return count
def get_depth(root):
if not root:
return 0
stack = [(root, 1)]
depth = 0
while stack:
node, curr_depth = stack.pop()
depth = max(depth, curr_depth)
if node.right:
stack.append((node.right, curr_depth + 1))
if node.left:
stack.append((node.left, curr_depth + 1))
return depth
def get_path(root, target):
if not root:
return []
stack = [(root, [root.val])]
while stack:
node, path = stack.pop()
if node.val == target:
return path
if node.right:
stack.append((node.right, path + [node.right.val]))
if node.left:
stack.append((node.left, path + [node.left.val]))
return []
```
其中,`build_tree` 函数根据先序遍历和中序遍历构建二叉树,`postorder_traversal` 函数输出后序遍历的结点序列,`get_leaf_nodes` 函数输出叶子结点,`count_nodes` 函数统计结点个数,`get_depth` 函数求二叉树的深度,`get_path` 函数输出指定结点的路径。
阅读全文
相关推荐
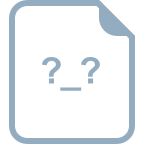















