c语言replace函数
时间: 2023-08-02 20:14:25 浏览: 57
C语言中没有内置的replace函数,但可以通过其他方式模拟实现。
一种实现方式是使用字符串拼接和循环,先找到要替换的子串的位置,然后将其前面和后面的字符串分别保存到两个新字符串中,再将替换的字符串拼接到中间,最后将两个新字符串拼接在一起即可。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
void replace(char *str, char *old, char *new) {
char result[100] = ""; // 保存替换后的字符串
int i, j, k, n = strlen(str), m = strlen(old);
for (i = 0; i < n;) {
j = i;
k = 0;
while (j < n && k < m && str[j] == old[k]) {
j++;
k++;
}
if (k == m) { // 找到了要替换的子串
strcat(result, new); // 拼接新字符串
i = j;
} else {
result[strlen(result)] = str[i]; // 拼接原字符串
i++;
}
}
strcpy(str, result); // 将替换后的字符串拷贝回原字符串
}
int main() {
char str[100] = "hello world";
replace(str, "world", "ChitChat");
printf("%s\n", str); // 输出 "hello ChitChat"
return 0;
}
```
需要注意的是,以上代码只是一个简单的示例,还有许多细节需要考虑,比如替换的字符串长度不同、替换后的字符串可能会超出原字符串长度等等。因此,在实际使用中需要更加严谨地处理。
相关推荐
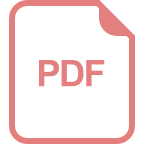














