c语言,用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/()。
时间: 2023-11-13 17:05:57 浏览: 51
可以使用栈来实现表达式求值。具体步骤如下:
1. 定义一个操作数栈(用于存储数字)和一个操作符栈(用于存储运算符)。
2. 从键盘读入一个表达式字符串,逐个字符进行处理。
3. 如果当前字符是数字,则将其转换为整型数值并压入操作数栈中。
4. 如果当前字符是左括号,直接将其压入操作符栈中。
5. 如果当前字符是右括号,则从操作符栈中弹出运算符进行运算,直至遇到左括号为止。
6. 如果当前字符是运算符,则比较其与操作符栈顶元素的优先级,如果当前运算符优先级较低,则从操作符栈中弹出运算符进行运算,直至当前运算符优先级不低于栈顶元素的优先级。
7. 最后,当表达式扫描完毕后,如果操作符栈中还有运算符,则依次弹出进行运算,最终得到表达式的值。
下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
typedef struct {
int top;
int data[100];
} Stack;
void initStack(Stack *s) {
s->top = -1;
}
int isEmpty(Stack *s) {
return s->top == -1;
}
void push(Stack *s, int x) {
s->data[++(s->top)] = x;
}
int pop(Stack *s) {
return s->data[(s->top)--];
}
int getTop(Stack *s) {
return s->data[s->top];
}
int priority(char op) {
switch(op) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
default:
return 0;
}
}
int calculate(int a, int b, char op) {
switch(op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return 0;
}
}
int evaluate(char *expr) {
Stack numStack, opStack;
initStack(&numStack);
initStack(&opStack);
while (*expr != '\0') {
if (isdigit(*expr) || (*expr == '-' && isdigit(*(expr+1)))) {
int num = 0, sign = 1;
if (*expr == '-') {
sign = -1;
++expr;
}
while (isdigit(*expr)) {
num = num * 10 + (*expr - '0');
++expr;
}
push(&numStack, sign * num);
} else if (*expr == '(') {
push(&opStack, '(');
++expr;
} else if (*expr == ')') {
while (getTop(&opStack) != '(') {
char op = (char)pop(&opStack);
int b = pop(&numStack);
int a = pop(&numStack);
push(&numStack, calculate(a, b, op));
}
pop(&opStack);
++expr;
} else if (*expr == '+' || *expr == '-' || *expr == '*' || *expr == '/') {
while (!isEmpty(&opStack) && priority(getTop(&opStack)) >= priority(*expr)) {
char op = (char)pop(&opStack);
int b = pop(&numStack);
int a = pop(&numStack);
push(&numStack, calculate(a, b, op));
}
push(&opStack, *expr);
++expr;
} else {
++expr;
}
}
while (!isEmpty(&opStack)) {
char op = (char)pop(&opStack);
int b = pop(&numStack);
int a = pop(&numStack);
push(&numStack, calculate(a, b, op));
}
return pop(&numStack);
}
int main() {
char expr[100];
printf("请输入算术表达式:\n");
scanf("%s", expr);
int result = evaluate(expr);
printf("表达式求值结果为:%d\n", result);
return 0;
}
```
注意:此处假设输入的表达式是合法的,未作错误处理。
相关推荐







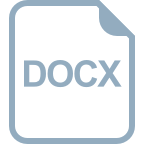
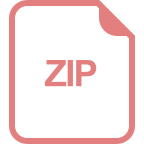
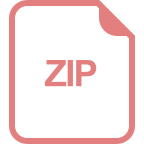
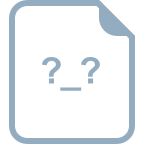
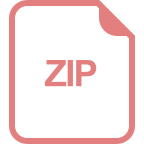