用c语言写一个扫雷小游戏
时间: 2023-12-29 08:51:00 浏览: 28
以下是一个简单的扫雷游戏的C语言代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define ROW 9
#define COL 9
#define TOTAL 10
void InitBoard(char board[][COL], int row, int col, char set);
void SetMine(char board[][COL], int row, int col, int total);
void PrintBoard(char board[][COL], int row, int col);
char GetMineCount(char board[][COL], int x, int y);
int main()
{
char board[ROW][COL];
int x, y, count = 0;
char result;
srand((unsigned int)time(NULL));
InitBoard(board, ROW, COL, ' ');
SetMine(board, ROW, COL, TOTAL);
PrintBoard(board, ROW, COL);
do {
printf("请输入坐标(格式:x y):");
scanf("%d %d", &x, &y);
result = GetMineCount(board, x, y);
if (result == '*') {
printf("很遗憾,你被炸了!\n");
break;
}
else {
board[x][y] = result;
PrintBoard(board, ROW, COL);
count++;
}
} while (count < ROW * COL - TOTAL);
if (count == ROW * COL - TOTAL) {
printf("恭喜你,扫雷成功!\n");
}
return 0;
}
// 初始化游戏面板
void InitBoard(char board[][COL], int row, int col, char set)
{
int i, j;
for (i = 0; i < row; i++) {
for (j = 0; j < col; j++) {
board[i][j] = set;
}
}
}
// 布雷
void SetMine(char board[][COL], int row, int col, int total)
{
int i, j, k;
for (k = 0; k < total; k++) {
do {
i = rand() % row;
j = rand() % col;
} while (board[i][j] == '*');
board[i][j] = '*';
}
}
// 计算某个位置周围雷的数量
char GetMineCount(char board[][COL], int x, int y)
{
if (board[x][y] == '*') {
return '*';
}
int count = 0, i, j;
for (i = x - 1; i <= x + 1; i++) {
for (j = y - 1; j <= y + 1; j++) {
if (i >= 0 && i < ROW && j >= 0 && j < COL && board[i][j] == '*') {
count++;
}
}
}
return count + '0';
}
// 打印游戏面板
void PrintBoard(char board[][COL], int row, int col)
{
int i, j;
printf(" ");
for (i = 0; i < col; i++) {
printf("%d ", i);
}
printf("\n");
for (i = 0; i < row; i++) {
printf("%d ", i);
for (j = 0; j < col; j++) {
printf("%c ", board[i][j]);
}
printf("\n");
}
}
```
运行后,游戏会显示如下:
```
0 1 2 3 4 5 6 7 8
0 *
1 *
2
3
4 * *
5
6
7
8
请输入坐标(格式:x y):
```
玩家输入坐标后,游戏会显示出该位置周围雷的数量,或者显示出“*”表示该位置为雷,如下:
```
0 1 2 3 4 5 6 7 8
0 1 2 * 1 1
1 1 2 * 1 1 1
2 1 1 1 1 1 1 1 1 1
3 1 1 1 1 1 1 1 1 1
4 1 * 1 1 * 1
5 2 2 2 1 1 2 2 2 2
6 * 2 1 1 1 *
7 2 2 1 1 1
8 1 1 1 1 1 1 1 1
请输入坐标(格式:x y):
```
玩家继续输入坐标,直到全部不是雷的位置都被翻开,或者翻开一个雷。
相关推荐











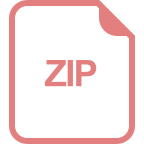
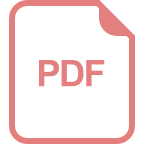