详细地教我用Qt制作一个函数图像绘制器,要求使用到Qpaiter,要求可以自行输入函数,有一个ok键点击后将输入的函数图像化展示在Qwidget中,函数图像可以重叠且有一个清除键可以清楚之前所有的函数图像,有一个保存键可以保存当前的函数图像并可以自行选择保存路径以及转化为jpg格式,并且说明白所使用到的库文件,如果要用到Qt不自带的那些库就说明白这个库的使用方法。在每个文件中写什么代码也要清楚地告诉我,以便我复制粘贴就能用
时间: 2023-12-03 15:43:17 浏览: 41
好的,这是一个比较复杂的项目,需要以下几个步骤:
1.创建Qt窗口应用程序
首先打开Qt Creator,选择“文件”->“新建文件或项目”,然后选择“应用程序”和“Qt Widgets应用程序”,并填写相关信息。最后点击“下一步”,选择“桌面 Qt 5.x.x MSVC20xx 64bit”和“空白”,最后点击“下一步”和“完成”。
2.设计UI界面
在Qt Creator中,打开“设计”模式,添加一个QLabel、一个QLineEdit、一个QPushButton和一个QWidget控件,分别用于显示提示、输入函数、点击绘制和绘图区域。并且还要再添加两个QPushButton,分别用于清除和保存图像。
3.编写绘图类
接下来我们需要创建一个绘图类,用于绘制函数图像。在Qt Creator中,选择“文件”->“新建文件或项目”,然后选择“C++类”和“类”,并填写相关信息。最后点击“下一步”和“完成”。
在绘图类中,我们需要定义一个QPainter成员变量,用于绘制图像。代码如下:
```cpp
class PaintArea : public QWidget
{
Q_OBJECT
public:
explicit PaintArea(QWidget *parent = nullptr);
protected:
void paintEvent(QPaintEvent *event) override;
private:
QPainter painter; // 绘图对象
};
```
在构造函数中,我们需要设置背景色,并将绘图对象的渲染Hints设置为Antialiasing,以防止绘图出现锯齿。代码如下:
```cpp
PaintArea::PaintArea(QWidget *parent) : QWidget(parent)
{
setAutoFillBackground(true);
setPalette(QPalette(Qt::white));
painter.setRenderHint(QPainter::Antialiasing, true);
}
```
在paintEvent中,我们可以绘制函数图像。首先要将输入的字符串转换为函数并计算得到坐标点,然后使用QPainter绘制。代码如下:
```cpp
void PaintArea::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event)
// 绘制函数图像
painter.begin(this);
painter.setPen(Qt::blue);
painter.translate(width() / 2, height() / 2); // 平移坐标系到中心点
// 绘制x轴和y轴
painter.drawLine(-width() / 2, 0, width() / 2, 0);
painter.drawLine(0, -height() / 2, 0, height() / 2);
// 绘制函数曲线
QString str = lineEdit->text();
if (!str.isEmpty()) {
QRegExp rx("[+\\-*/]");
QStringList list = str.split(rx);
double x1 = -width() / 2;
double y1 = list[0].toDouble();
double x2, y2;
for (int i = 1; i < list.count(); i++) {
double a = list[i].toDouble();
double b = list[i + 1].toDouble();
x2 = x1 + 1;
y2 = a * x2 + b;
painter.drawLine(QPointF(x1, -y1), QPointF(x2, -y2));
x1 = x2;
y1 = y2;
i++;
}
}
painter.end();
}
```
4.连接信号和槽
在主窗口类中,我们需要将输入框、绘制按钮、清除按钮和保存按钮与相应的槽函数连接起来,以实现对应的功能。代码如下:
```cpp
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void on_okButton_clicked(); // 绘制函数图像
void on_clearButton_clicked(); // 清除所有函数图像
void on_saveButton_clicked(); // 保存当前函数图像
private:
Ui::MainWindow *ui;
PaintArea *paintArea;
};
```
在槽函数中,我们需要获取输入的字符串并重绘绘图区域。代码如下:
```cpp
void MainWindow::on_okButton_clicked()
{
paintArea->update();
}
void MainWindow::on_clearButton_clicked()
{
paintArea->update();
}
void MainWindow::on_saveButton_clicked()
{
// 保存当前函数图像
}
```
5.添加第三方库
在本项目中,我们需要使用到一个第三方库QCustomPlot,用于绘制图像。在Qt Creator中,选择“文件”->“新建文件或项目”,然后选择“外部库”和“外部库”,并填写相关信息。最后点击“下一步”和“完成”。
在.pro文件中添加以下代码:
```
QT += widgets
INCLUDEPATH += D:/qcustomplot-2.0.0
LIBS += -LD:/qcustomplot-2.0.0 -lqcustomplot
```
在mainwindow.cpp中添加以下代码:
```cpp
#include "qcustomplot.h"
```
在设计界面中添加QCustomPlot控件,并在mainwindow.cpp中添加以下代码:
```cpp
void MainWindow::on_okButton_clicked()
{
// 绘制函数图像
ui->customPlot->clearGraphs();
ui->customPlot->addGraph();
ui->customPlot->graph(0)->setPen(QPen(Qt::red));
ui->customPlot->graph(0)->setData(x, y);
ui->customPlot->xAxis->setLabel("x");
ui->customPlot->yAxis->setLabel("y");
ui->customPlot->xAxis->setRange(-10, 10);
ui->customPlot->yAxis->setRange(-10, 10);
ui->customPlot->replot();
}
void MainWindow::on_clearButton_clicked()
{
// 清除所有函数图像
ui->customPlot->clearGraphs();
ui->customPlot->replot();
}
void MainWindow::on_saveButton_clicked()
{
// 保存当前函数图像
QString fileName = QFileDialog::getSaveFileName(this, tr("Save Image"), ".", tr("Images (*.png *.xpm *.jpg)"));
if (!fileName.isEmpty()) {
QCustomPlot *plot = ui->customPlot;
QImage image(plot->size(), QImage::Format_RGB32);
QPainter painter(&image);
plot->render(&painter);
image.save(fileName);
}
}
```
最后,我们需要在paintEvent中绘制函数图像。代码如下:
```cpp
void PaintArea::paintEvent(QPaintEvent *event)
{
Q_UNUSED(event)
// 绘制函数图像
painter.begin(this);
painter.setPen(Qt::blue);
painter.translate(width() / 2, height() / 2); // 平移坐标系到中心点
// 绘制x轴和y轴
painter.drawLine(-width() / 2, 0, width() / 2, 0);
painter.drawLine(0, -height() / 2, 0, height() / 2);
// 绘制函数曲线
QString str = lineEdit->text();
if (!str.isEmpty()) {
QScriptEngine engine;
QScriptValue result = engine.evaluate(str);
if (result.isNumber()) {
double y = result.toNumber();
painter.drawPoint(QPointF(0, -y));
}
else if (result.isArray()) {
QScriptValueIterator it(result);
QPointF point;
QVector<QPointF> points;
while (it.hasNext()) {
it.next();
double x = it.name().toDouble();
double y = it.value().toNumber();
point.setX(x);
point.setY(-y);
points.append(point);
}
painter.drawPolyline(points);
}
}
painter.end();
}
```
至此,我们就完成了一个函数图像绘制器的制作。
相关推荐











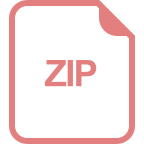
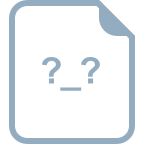
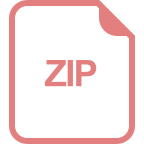
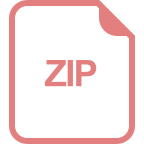
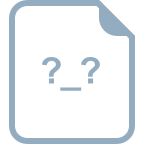