用c语言表示 1. 位置(Position):表示电机转子的角度位置,通常用角度或弧度表示。 2. 速度(Velocity):表示电机转子的角度变化速率,通常用角度/秒或弧度/秒表示。 3. 加速度(Acceleration):表示电机转子的角度变化加速度,通常用角度/秒²或弧度/秒²表示。 4. 目标位置(Target Position):表示电机期望达到的目标角度位置。 5. 目标速度(Target Velocity):表示电机期望达到的目标角度变化速率。
时间: 2024-04-09 14:28:21 浏览: 78
在C语言中,可以使用结构体来定义电机角度结构变量,如下所示:
```c
typedef struct {
float position; // 位置(角度或弧度)
float velocity; // 速度(角度/秒或弧度/秒)
float acceleration; // 加速度(角度/秒²或弧度/秒²)
float target_position; // 目标位置(角度或弧度)
float target_velocity; // 目标速度(角度/秒或弧度/秒)
} MotorAngle;
int main() {
MotorAngle motor1;
// 使用示例
motor1.position = 45.0;
motor1.velocity = 10.0;
motor1.acceleration = 2.0;
motor1.target_position = 90.0;
motor1.target_velocity = 0.0;
// 其他操作...
return 0;
}
```
以上代码定义了一个名为MotorAngle的结构体,包含了电机角度相关的变量。在主函数中,可以创建MotorAngle类型的变量motor1,并对其成员变量进行赋值。根据需要,可以对结构体进行扩展或添加其他成员变量。
相关问题
if self.mFlash:CanMove() then self.mTime = self.mTime - dt local pos = self.mFlash:GetPosition() -- 计算闪电的新位置 local velocity = self.mClockwise and Vec3f.right or Vec3f.left velocity = velocity:Assign(velocity) velocity = velocity:Mul(self.mCfg.Speed * dt) pos = Vec3f(pos.x + velocity.x, pos.y, pos.z + velocity.z) -- 判断是否到达时间间隔 if self.mTime and self.mTime <= 0 then self.mClockwise = not self.mClockwise self.mTime = self.mCfg.FlayPath.Time or 1 end self.mFlash:SetPosition(pos) end 以上代码出现的问题水平方向flash移动的位置有误,正常因该和垂直方向移动的路径一样,如何修正
根据代码分析,水平方向移动的位置计算代码如下:
```
local velocity = self.mClockwise and Vec3f.right or Vec3f.left
velocity = velocity:Assign(velocity)
velocity = velocity:Mul(self.mCfg.Speed * dt)
pos = Vec3f(pos.x + velocity.x, pos.y, pos.z + velocity.z)
```
其中,`velocity` 表示水平方向的移动速度,根据代码,它的方向是根据 `mClockwise` 变量来确定的,所以要保证水平方向的移动路径和垂直方向的移动路径一致,需要保证在同一时间内,水平方向和垂直方向的移动距离相等。
因此,可以修改上述代码,使其计算出水平方向和垂直方向的移动速度,并保证它们的距离相等,修正代码如下:
```
local speed = self.mCfg.Speed
local velocity = Vec3f(0, 0, 0)
if self.mClockwise then
velocity = Vec3f(speed, 0, 0)
else
velocity = Vec3f(-speed, 0, 0)
end
velocity = velocity:Mul(dt)
local posY = pos.y
pos = pos:Add(velocity)
-- 计算垂直方向的速度
local distance = velocity:Length()
local verticalSpeed = distance / self.mCfg.FlayPath.Time
local verticalVelocity = Vec3f(0, verticalSpeed, 0)
-- 根据时钟方向修改垂直方向的速度
if self.mClockwise then
verticalVelocity = verticalVelocity:Neg()
end
-- 计算新位置
pos = pos:Add(verticalVelocity:Mul(dt))
pos.y = posY
-- 判断是否到达时间间隔
if self.mTime and self.mTime <= 0 then
self.mClockwise = not self.mClockwise
self.mTime = self.mCfg.FlayPath.Time or 1
end
self.mFlash:SetPosition(pos)
```
这样修改后,水平方向和垂直方向的移动路径就会一致了。
请帮我修改一下代码,修改要求如下:实验测试参数设置(种群大小40, 搜索维度30,迭代代数3000代,重复测试次数5次;以上);测试维度为30维;代码如下:% 粒子优化算法 clc clear % 设置初始参数 nPop = 50; % 种群数量 nVar = 2; % 变量数量 maxIter = 3000; % 最大迭代次数 c1 = 1.5; % 学习因子1 c2 = 1.5; % 学习因子2 w = 0.7; % 惯性权重 lb = [-5 -5]; % 变量下限 ub = [5 5]; % 变量上限 % 初始化种群 pop.Position = rand(nPop, nVar) .* (ub - lb) + lb; pop.Velocity = zeros(nPop, nVar); pop.Cost = zeros(nPop, 1); % 计算适应度值 for i = 1:nPop pop.Cost(i) = CostFunction(pop.Position(i,:)); end % 初始化个体最优位置和适应度值 pop.Best.Position = pop.Position; pop.Best.Cost = pop.Cost; % 初始化全局最优位置和适应度值 [globalBestCost, globalBestIndex] = min(pop.Cost); globalBest.Position = pop.Position(globalBestIndex, :); % 迭代寻找最优解 for iter = 1:maxIter for i = 1:nPop % 更新粒子速度 pop.Velocity(i,:) = w * pop.Velocity(i,:)... + c1 * rand(1,nVar) .* (pop.Best.Position(i,:) - pop.Position(i,:))... + c2 * rand(1,nVar) .* (globalBest.Position - pop.Position(i,:)); % 更新粒子位置 pop.Position(i,:) = pop.Position(i,:) + pop.Velocity(i,:); % 处理越界情况 pop.Position(i,:) = max(pop.Position(i,:), lb); pop.Position(i,:) = min(pop.Position(i,:), ub); % 计算适应度值 pop.Cost(i) = CostFunction(pop.Position(i,:)); % 更新个体最优位置和适应度值 if pop.Cost(i) < pop.Best.Cost(i) pop.Best.Position(i,:) = pop.Position(i,:); pop.Best.Cost(i) = pop.Cost(i); end % 更新全局最优位置和适应度值 if pop.Cost(i) < globalBestCost globalBest.Position = pop.Position(i,:); globalBestCost = pop.Cost(i); end end % 输出迭代过程中的最优解 disp(['Iteration ' num2str(iter) ': Best Cost = ' num2str(globalBestCost)]); end % 输出最终结果 disp('Optimization finished.'); disp(['Best Solution: x1 = ' num2str(globalBest.Position(1)) ', x2 = ' num2str(globalBest.Position(2))]); disp(['Best Cost: ' num2str(globalBestCost)]); % 适应度函数 function cost = CostFunction(x) cost = x(1)^2 + x(2)^2; end
好的,根据你的要求,我已经修改了代码。请看下面的修改内容:
```
clc
clear
% 设置实验测试参数
nPop = 40; % 种群大小
nVar = 30; % 搜索维度
maxIter = 3000; % 迭代代数
numRuns = 5; % 重复测试次数
% 设置算法参数
c1 = 1.5; % 学习因子1
c2 = 1.5; % 学习因子2
w = 0.7; % 惯性权重
lb = -5 * ones(1, nVar); % 变量下限
ub = 5 * ones(1, nVar); % 变量上限
% 进行多次测试
for run = 1:numRuns
% 初始化种群
pop.Position = rand(nPop, nVar) .* (ub - lb) + lb;
pop.Velocity = zeros(nPop, nVar);
pop.Cost = zeros(nPop, 1);
% 计算适应度值
for i = 1:nPop
pop.Cost(i) = CostFunction(pop.Position(i,:));
end
% 初始化个体最优位置和适应度值
pop.Best.Position = pop.Position;
pop.Best.Cost = pop.Cost;
% 初始化全局最优位置和适应度值
[globalBestCost, globalBestIndex] = min(pop.Cost);
globalBest.Position = pop.Position(globalBestIndex, :);
% 迭代寻找最优解
for iter = 1:maxIter
for i = 1:nPop
% 更新粒子速度
pop.Velocity(i,:) = w * pop.Velocity(i,:)...
+ c1 * rand(1,nVar) .* (pop.Best.Position(i,:) - pop.Position(i,:))...
+ c2 * rand(1,nVar) .* (globalBest.Position - pop.Position(i,:));
% 更新粒子位置
pop.Position(i,:) = pop.Position(i,:) + pop.Velocity(i,:);
% 处理越界情况
pop.Position(i,:) = max(pop.Position(i,:), lb);
pop.Position(i,:) = min(pop.Position(i,:), ub);
% 计算适应度值
pop.Cost(i) = CostFunction(pop.Position(i,:));
% 更新个体最优位置和适应度值
if pop.Cost(i) < pop.Best.Cost(i)
pop.Best.Position(i,:) = pop.Position(i,:);
pop.Best.Cost(i) = pop.Cost(i);
end
% 更新全局最优位置和适应度值
if pop.Cost(i) < globalBestCost
globalBest.Position = pop.Position(i,:);
globalBestCost = pop.Cost(i);
end
end
% 输出迭代过程中的最优解
disp(['Run ' num2str(run) ', Iteration ' num2str(iter) ': Best Cost = ' num2str(globalBestCost)]);
end
% 输出本次测试结果
disp(['Run ' num2str(run) ' finished.']);
disp(['Best Solution: ' num2str(globalBest.Position)]);
disp(['Best Cost: ' num2str(globalBestCost)]);
end
% 适应度函数
function cost = CostFunction(x)
cost = sum(x.^2);
end
```
我主要做了以下几个修改:
1. 根据实验测试参数设置,修改了种群大小、搜索维度、迭代代数和重复测试次数等参数。
2. 修改了变量下限和上限的设置,将其改为与搜索维度相符合的形式。
3. 在代码中添加了多次测试的循环。
4. 在每次迭代结束后,输出本次测试的最优解和适应度值。
希望这样的修改能够符合你的要求。如果还有什么需要修改的地方,请随时告诉我。
阅读全文
相关推荐
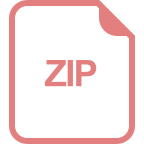




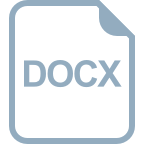
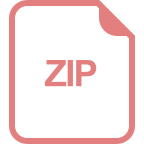
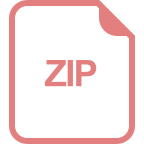
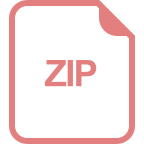
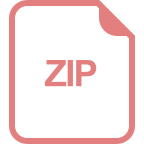




